Mastering Performance Testing with Locust: A Practical Guide
Performance Testing with Locust – Introduction
Locust is a Python-based open-source load testing tool that allows you to design test scenarios. It is scalable, user-friendly, and delivers real-time information. This blog post will walk you through the process of utilizing Locust, including examples of test implementation. The purpose is to help you grasp Locust’s power and how to use it for your performance testing needs.
Understanding Locust
Locust is event-based and uses coroutines instead of threads, which makes it lightweight and capable of handling thousands of users on a single machine. It allows you to write test cases as code, making them highly customizable and maintainable. This flexibility is one of the key advantages of Locust. It’s also distributed and scalable, meaning you can simulate millions of users by running tests on multiple machines.
Setting Up Locust
Locust is Python-based, so you’ll need Python installed on your machine. You can check if Python is installed by running the following command in your terminal:
python --version
If Python is installed, you should see a version number. If not, you’ll need to install Python first.
Once Python is installed, you can install Locust using pip, Python’s package installer. Run the following command in your terminal:
pip install locust
After the installation is complete, you can verify that Locust was installed correctly by running:
locust --version
You should see the version of Locust that was installed.
Once Locust is installed, you can start writing your test scripts. Locust scripts are plain Python files, which means you can use any Python libraries or modules in your tests. This opens up a world of possibilities for complex and powerful load tests.
Creating Your First Load Test
In Locust, user behavior is defined in a Python class. Here’s a simple example:
from locust import HttpUser, task, between
class WebsiteUser(HttpUser):
wait_time = between(5, 15)
@task
def index_page(self):
self.client.get("/")
@task
def about_page(self):
self.client.get("/about")
In this script, WebsiteUser represents a user. The wait_time attribute determines the wait time between tasks, and the @task decorator marks methods as tasks.
Running the Load Test
To run the test, save the script to a file (e.g., locustfile.py), then run the locust command in your terminal:
locust -f locustfile.py
This starts the Locust web interface. Here, you can specify the number of users to simulate and the spawn rate.
Analyzing Test Results
After running a test, it’s important to analyze the results to understand how your system performed. Locust provides several ways to do this. You can view real-time statistics in the web interface, or you can export the results to a CSV file for further analysis. When analyzing the results, look for any slow requests or failures that might indicate a performance issue.
Real-World Example
Let’s consider a scenario where you’re testing a web application. You might define a user class with methods for logging in, browsing items, adding an item to the cart, and checking out. You would then run a test simulating hundreds or thousands of these users to see how your application performs under load. This kind of realistic, code-based testing is where Locust shines.
from locust import HttpUser, task, between
class Shopper(HttpUser):
wait_time = between(1, 2)
@task
def view_product(self):
self.client.get("/product")
@task
def add_to_cart(self):
self.client.post("/cart", json={"product_id": 1})
@task
def checkout(self):
self.client.post("/checkout")
Python Performance Testing – Summary
Locust is a powerful tool for performance testing that stands out due to its flexibility and scalability. Its ability to simulate thousands of users with realistic behavior makes it an excellent choice for testing web applications. With its Python-based scripting, it offers a high degree of customization, allowing you to tailor your tests to your specific needs.
In this guide, we’ve walked through the basics of setting up Locust, creating a simple load test, running the test, and analyzing the results. We’ve also looked at a real-world example of testing an e-commerce website. However, this is just scratching the surface of what you can do with Locust.
Beyond the basics, Locust supports more advanced features like test distribution across multiple machines, complex user behavior modeling, and integration with other systems through its Python-based scripting. By mastering these capabilities, you can build more realistic tests that better represent your users’ behavior, helping you build applications that can stand up to real-world conditions.
Remember, the key to effective performance testing is understanding your users’ behavior and your system’s performance requirements. With this understanding and the power of Locust, you can ensure your applications are ready to handle whatever load your users throw at them. Happy testing!
Looking for IT Project Outsourcing? Check out our offer!
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us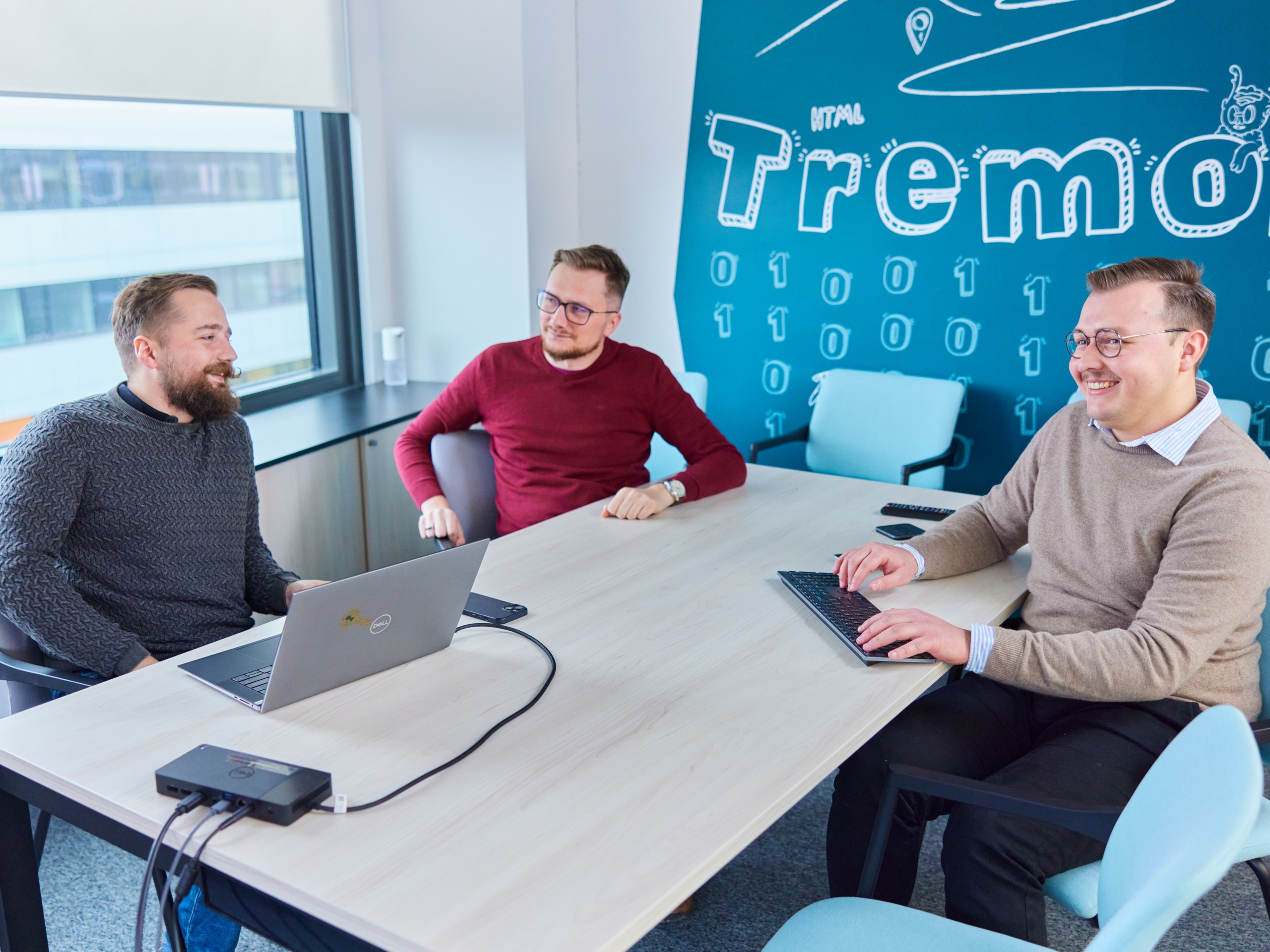
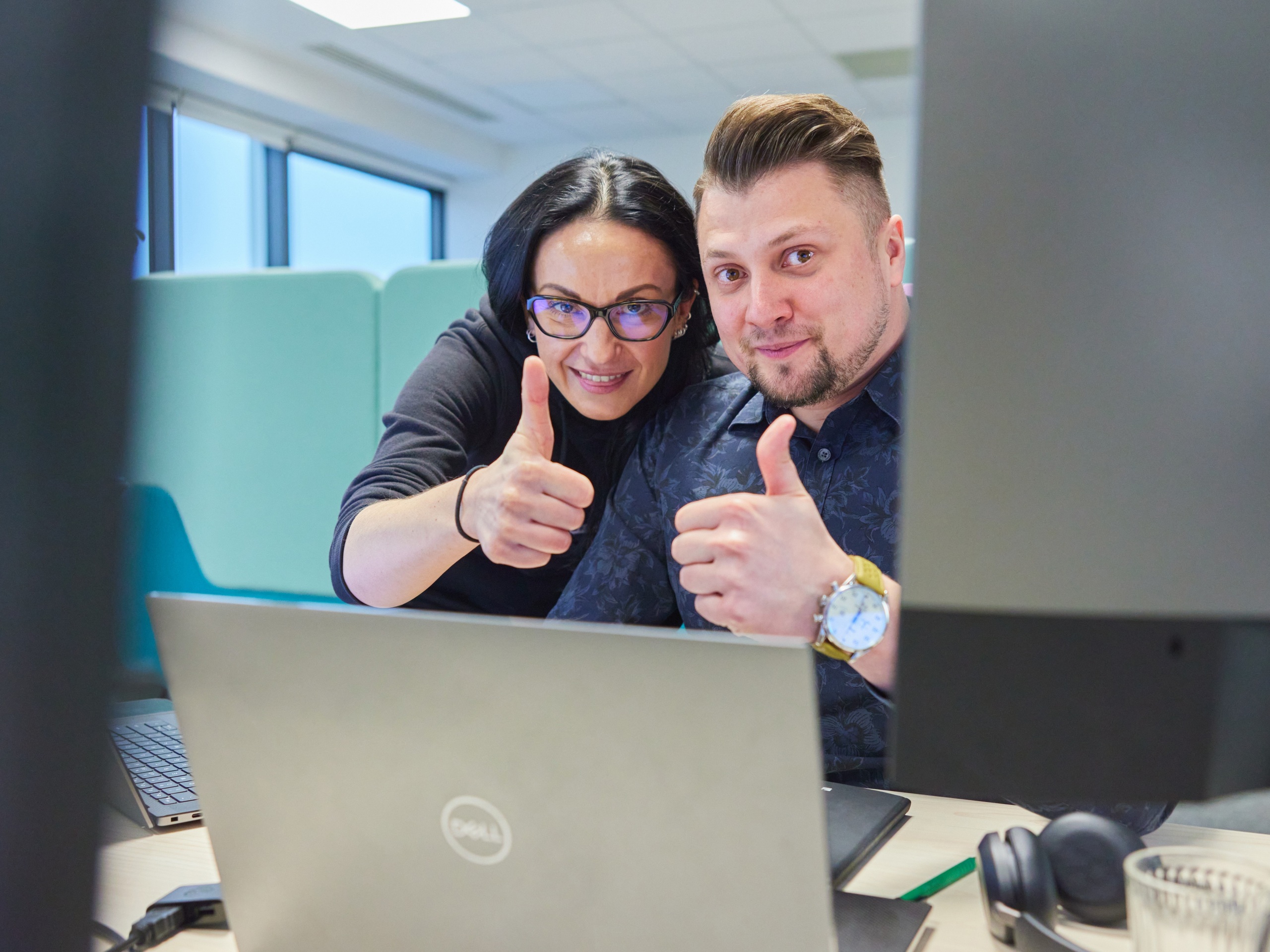
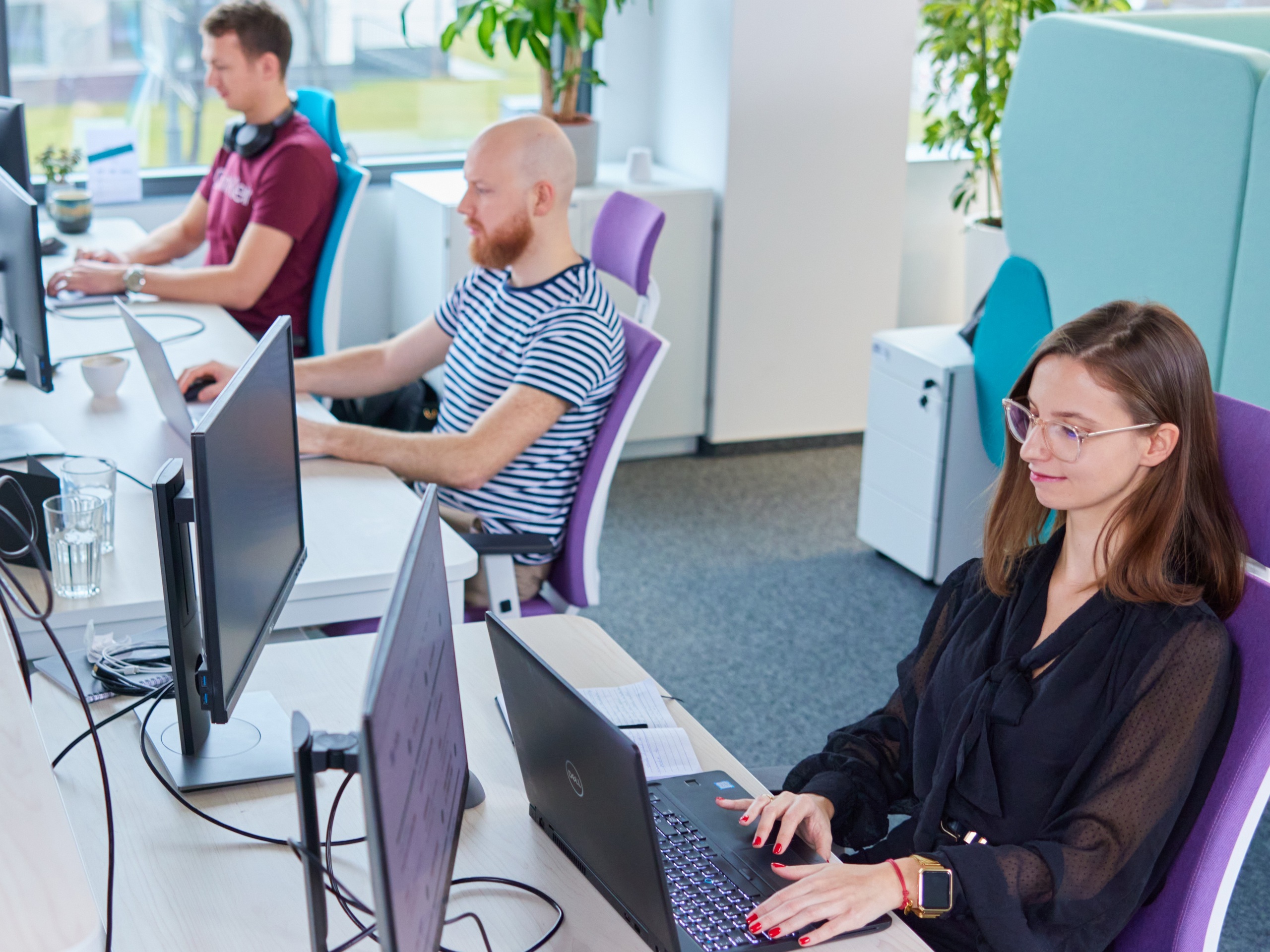
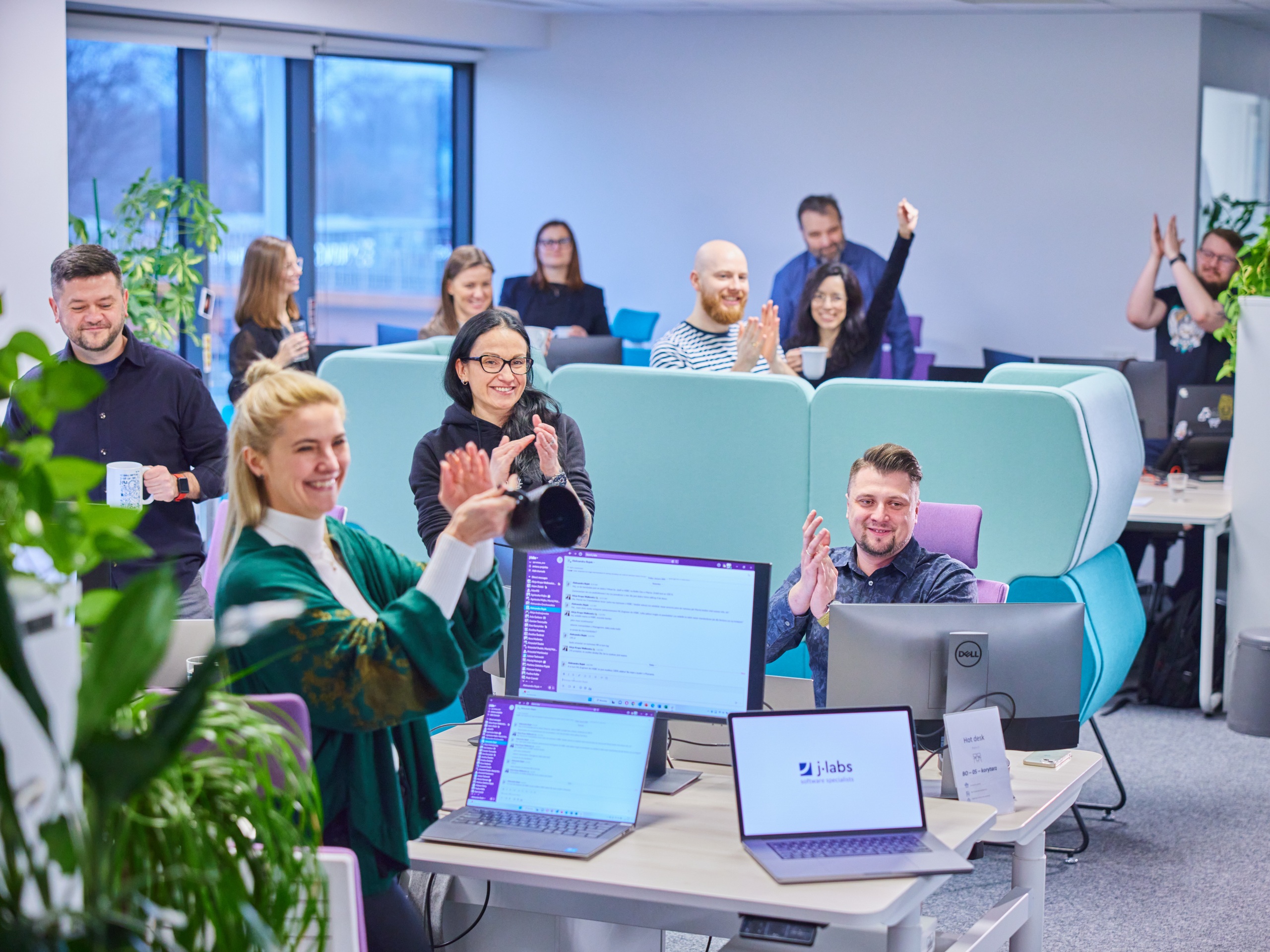