Streamlining Test Reporting with Xray: Leveraging Selenium, Java and Jenkins
Introduction
Automated testing has become an essential aspect of maintaining software quality and decreasing time-to-market in today’s software development ecosystem. Teams frequently rely on sophisticated solutions like Xray, which interfaces smoothly with popular frameworks like Selenium and Jenkins, to successfully manage and report test results. In this article, we’ll look at how to report test results in Xray with Selenium and Java for test automation and Jenkins for continuous integration.
Setting up Xray Test Automation with Selenium and Java
Before diving into test reporting, let’s briefly touch upon the setup required to automate tests using Selenium and Java. Selenium is a widely-used open-source testing framework that provides a rich set of tools and libraries for browser automation. Here’s an example of a simple login test written in Java using Selenium:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class LoginTest {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
WebElement usernameField = driver.findElement(By.id("username"));
usernameField.sendKeys("myUsername");
WebElement passwordField = driver.findElement(By.id("password"));
passwordField.sendKeys("myPassword");
WebElement loginButton = driver.findElement(By.id("login-button"));
loginButton.click();
// Perform assertions and further test steps as needed
driver.quit();
}
}
Introducing Xray for Test Management and Reporting
Jenkins is a popular open-source automation server widely used for continuous integration and delivery. It can be leveraged to schedule and execute automated test runs, including test reporting with Xray. To achieve this, you need to define a Jenkins pipeline, often referred to as a Jenkinsfile, which outlines the steps to build, test, and report results. Here’s an example of a simplified Jenkinsfile for running automated tests and reporting results to Xray:
pipeline {
agent any
stages {
stage('Build') {
steps {
// Perform build steps if necessary
}
}
stage('Test') {
steps {
sh 'mvn test' // Run the automated tests using Maven or a specific test runner
}
}
stage('Report Results') {
steps {
xrayImportResults(
testResults: '**/target/surefire-reports/*.xml', // Specify the location of test result files
testExecutionKey: 'PROJ-123', // Unique identifier for the test execution in Xray
projectKey: 'PROJ' // Key of the Xray project where results should be imported
)
}
}
}
}
Importing Test Results to Xray
Once the automated tests have been executed successfully using Jenkins, the next step is to import the test results into Xray for comprehensive reporting and analysis. The ‘xrayImportResults’ step in the Jenkinsfile is responsible for this crucial task. When the ‘xrayImportResults’ step is triggered, it takes the generated test result files, typically in the XML format (e.g., generated by Surefire), and imports them into Xray. This step requires specifying the location of the test result files using a file path or a wildcard pattern. For example, ‘*/target/surefire-reports/.xml’ specifies that all XML files in the ‘target/surefire-reports’ directory should be imported.
In addition to the test result files, the ‘xrayImportResults’ step also requires two important parameters: the test execution key and the project key. The test execution key is a unique identifier in Xray that associates the imported results with a specific test execution. It helps in tracking and comparing results over time. The project key represents the key of the Xray project where the results should be imported.
By providing the necessary parameters, the ‘xrayImportResults’ step triggers the process of importing the test results into Xray. Xray intelligently parses the XML files, extracting relevant information such as test names, statuses, and duration. It updates the corresponding test execution in Xray, reflecting the latest results.
Once the test results are imported, Xray provides a comprehensive view of the test execution’s status. Teams can easily identify passed, failed, or skipped tests, along with detailed information about each test case. Xray’s intuitive interface allows users to drill down into individual test cases, view associated defects, and track test coverage.
By importing test results into Xray, teams can harness its powerful reporting capabilities. Xray offers a range of reports, including test execution summaries, trends, traceability matrices, and defect tracking. These reports provide valuable insights into the quality of the software, allowing teams to make data-driven decisions, prioritize testing efforts, and address any issues promptly.
Analyzing Test Results in Xray
Once the test results are imported into Xray, teams can take advantage of its rich reporting capabilities. Xray provides various views and metrics to analyze test execution results, track progress, identify trends, and generate comprehensive reports. Teams can easily visualize the test coverage, identify failing tests, and gain insights into the overall health of their software.
Summary
Effective test reporting is crucial for maintaining a high level of software quality. By integrating Selenium, Java, Jenkins, and Xray, teams can streamline the process of running automated tests, generating comprehensive reports, and tracking the status of test executions. Xray’s seamless integration with popular automation tools like Selenium, combined with Jenkins’ powerful pipeline capabilities, provides a robust solution for teams looking to improve their test reporting workflows and ensure efficient test management. With Xray, teams can easily analyze test results, identify issues, and make data-driven decisions to deliver high-quality software.
Read also blog post about Angular signals!
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us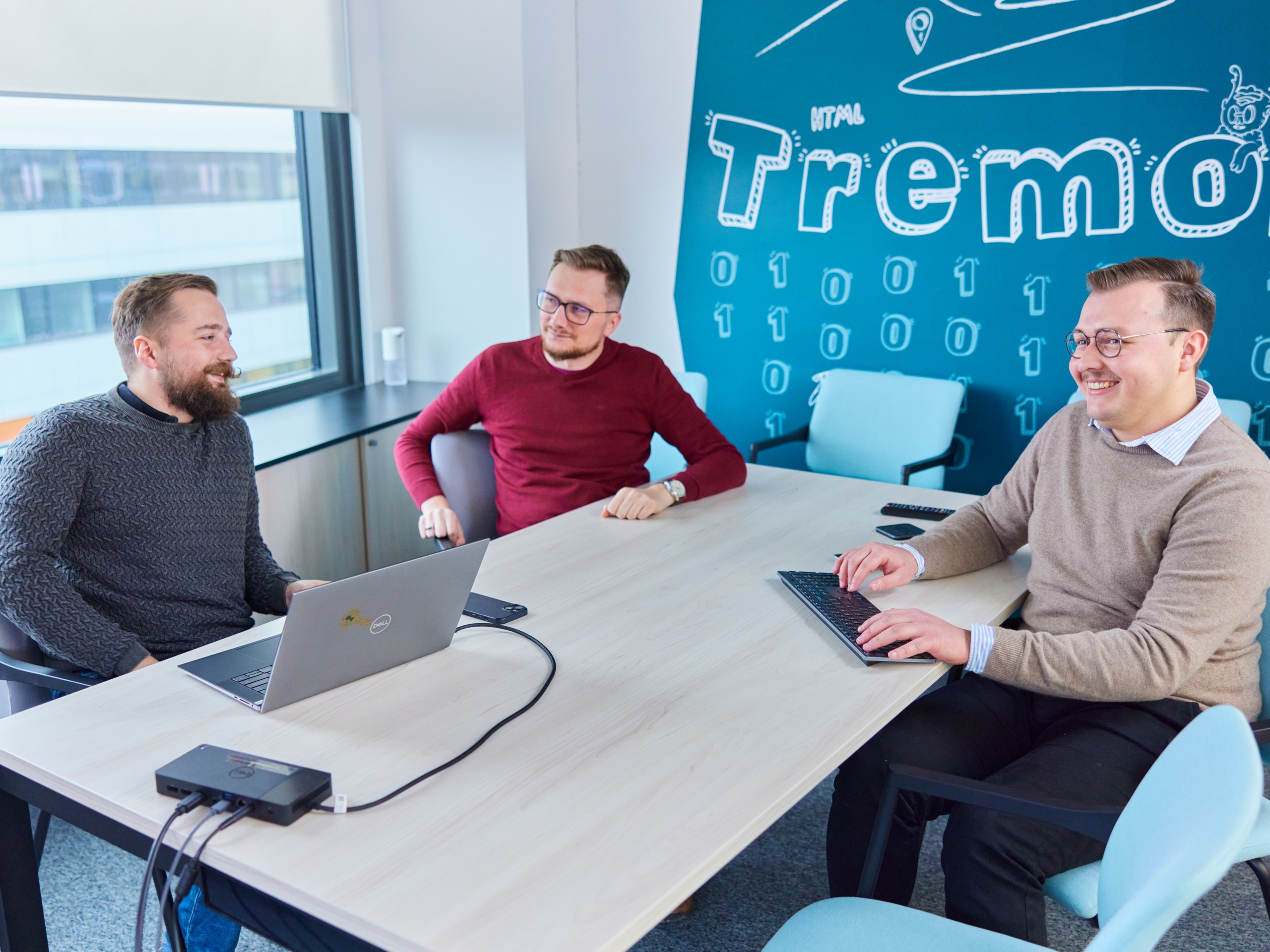
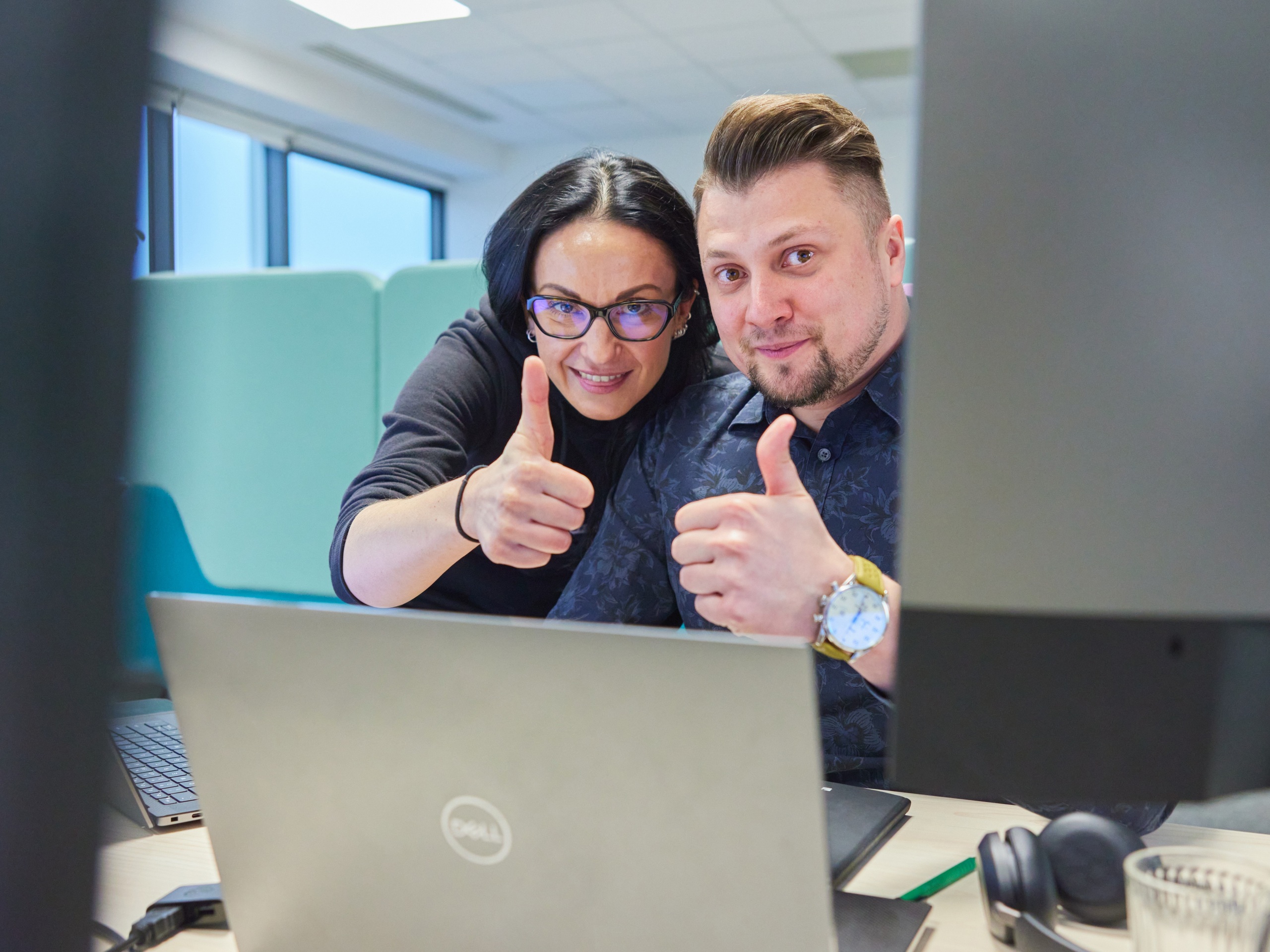
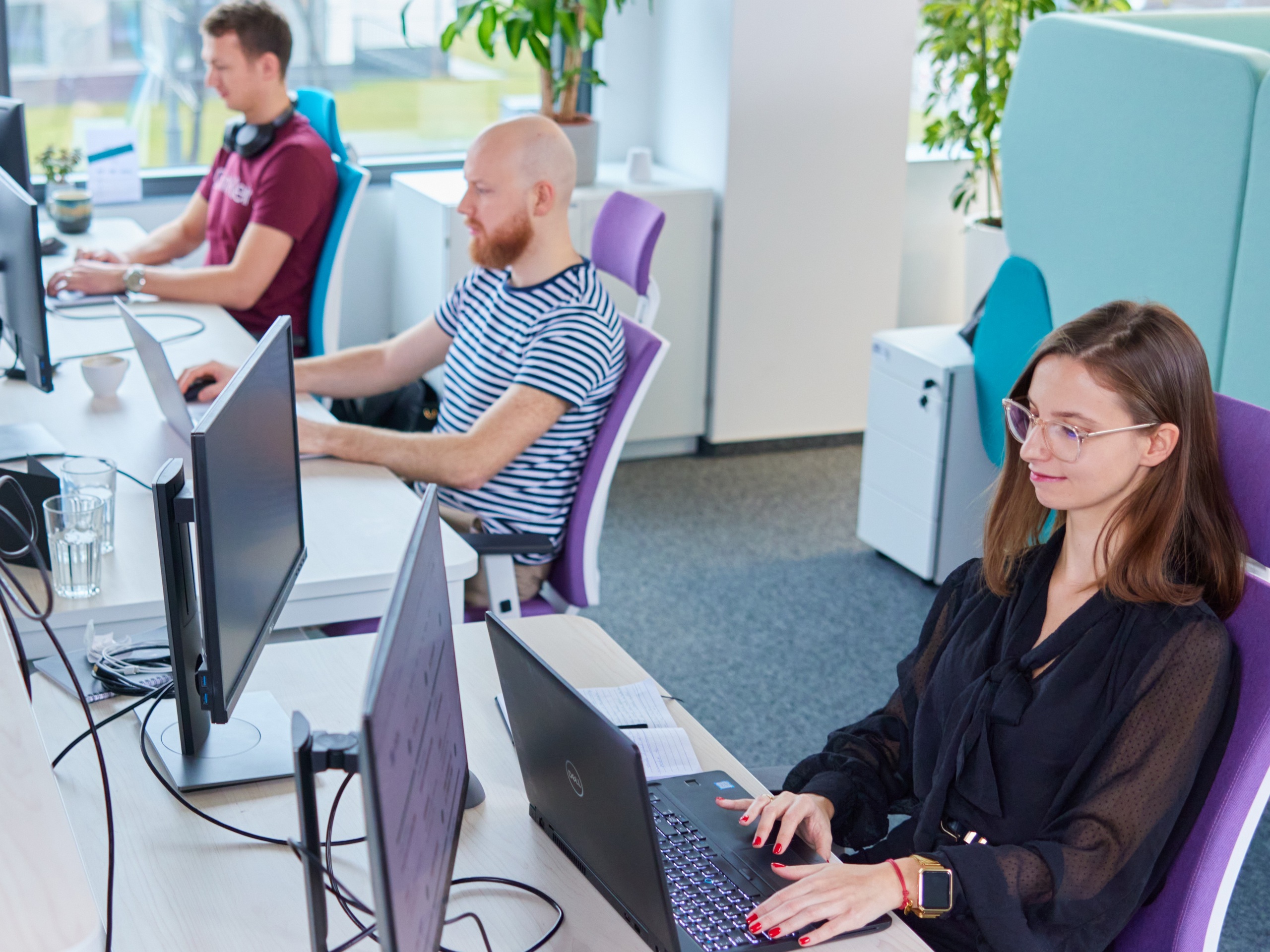
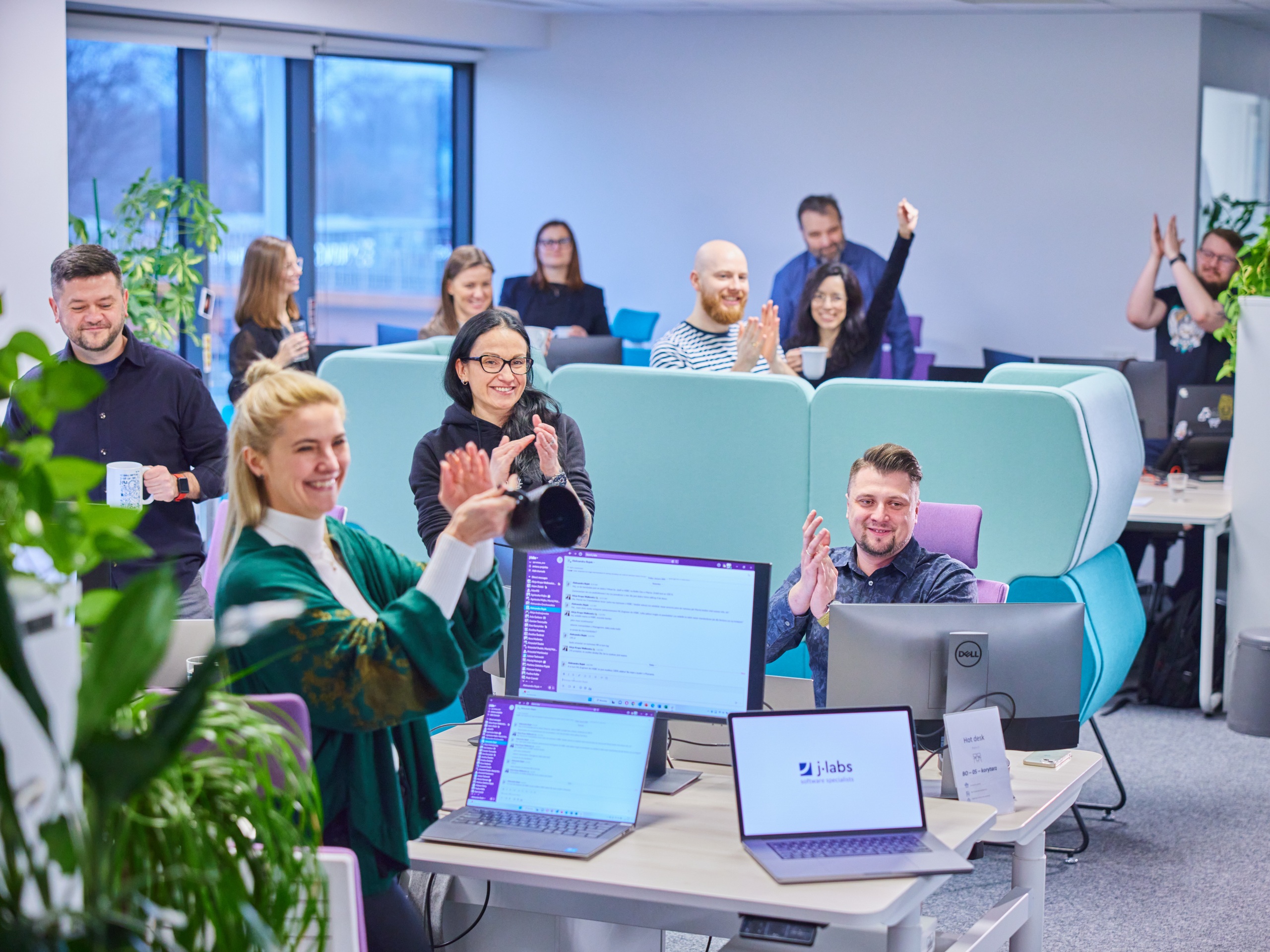