How to lock objects in JavaScript
JavaScript Lock Objects – Introdution
JavaScript provides various methods to control the behavior and mutability of objects. Among these methods, Object.preventExtensions
, Object.seal
, and Object.freeze
are particularly useful when it comes to managing object properties. These methods allow developers to set different levels of immutability and restrict the addition or deletion of properties.
Object.preventExtensions
The Object.preventExtensions()
method is used in JavaScript to prevent any further properties from being added to an object. When an object is marked as non-extensible, you cannot add new properties to it, although you can still modify or delete the existing properties.
This can be useful in scenarios where you want to enforce a specific set of properties and prevent accidental additions or modifications to the object’s structure. It helps in maintaining data integrity and prevents unintended changes to important object properties. In some cases, when an object is marked as non-extensible, JavaScript engines can make certain optimizations, assuming that the object’s structure will not change. This can result in performance improvements during property access or iteration, as the engine doesn’t have to check for new properties being added.
const user = {
name: "John Doe",
age: "25"
};
Object.preventExtensions(user);
Object.isExtensible(user); // false
user.age = 30; // Modification is allowed
user.email = "johndoe@example.com"; // Adding new property is ignored or throws an error
delete user.name; // Deletion is allowed
Object.seal
The Object.seal()
method in JavaScript is used to seal an object, which means it prevents new properties from being added to the object and marks all existing properties as non-configurable. It’s important to note that Object.seal()
does not make an object’s properties read-only. You can still modify the values of existing properties, but you cannot add, delete, or change the configurability of properties.
By sealing an object, you ensure that its structure remains fixed. This can be useful when you want to prevent any additions or deletions of properties, while still allowing modifications to the existing property values. It helps maintain the integrity and the expected structure of an object.
const user = {
name: "John Doe",
age: "25"
};
Object.seal(user);
Object.isSealed(user); // true
user.age = 30; // Modification is allowed
user.email = "johndoe@example.com"; // Adding new property is ignored or throws an error
delete user.name; // Deletion is ignored or throws an error
Object.freeze
The Object.freeze()
method in JavaScript is used to freeze an object, making it completely immutable. It prevents new properties from being added, existing properties from being modified, and properties from being deleted. Once an object is frozen, its state cannot be changed.
const user = {
name: "John Doe",
age: "25",
address: {
street: "Main St 1"
}
};
Object.freeze(user);
Object.isFrozen(user); // true
user.age = 30; // Modification is ignored or throws an error
user.email = "johndoe@example.com"; // Adding new property is ignored or throws an error
user.address.city = "London"; // Adding new nested property is allowed
delete user.name; // Deletion is ignored or throws an error
By making an object immutable, you reduce the risk of unauthorized modifications to its properties. This can be particularly relevant when dealing with sensitive data or objects that should not be tampered with. This is particularly useful in scenarios where an object is shared across different parts of an application or passed as an argument to functions, and freezing it can help ensure that its properties remain consistent and unchanged.
Nested properties
It’s important to note that all the methods above provide shallow immutability. This means that while the properties of the locked object cannot be added, deleted or modified, depending on the used method, if a property refers to an object, the properties of that object can still be changed unless they are also locked.
const user = {
address: {
street: "Main St 1"
}
};
Object.freeze(user);
Object.isFrozen(user); // true
Object.isFrozen(user.address); // false
user.address.city = "London"; // Adding new nested property is allowed
Strict mode
Strict mode can help you identify potential issues with code that attempts to modify a locked object. When you attempt to prevent extensions, seal, or freeze an object in strict mode, JavaScript will throw an error, usually a TypeError, indicating that the operation is not allowed. However, if you attempt to do the same not in strict mode, the code will fail silently.
Lock in JavaScript – Conclusion
Object.freeze
, Object.preventExtensions
, and Object.seal
are powerful tools in JavaScript for managing object properties and enforcing immutability. These methods allow developers to control the mutability of objects at different levels, depending on their specific requirements. By using these methods, you can ensure data integrity, enforce object structure, and reduce unexpected behaviors in your JavaScript code.
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us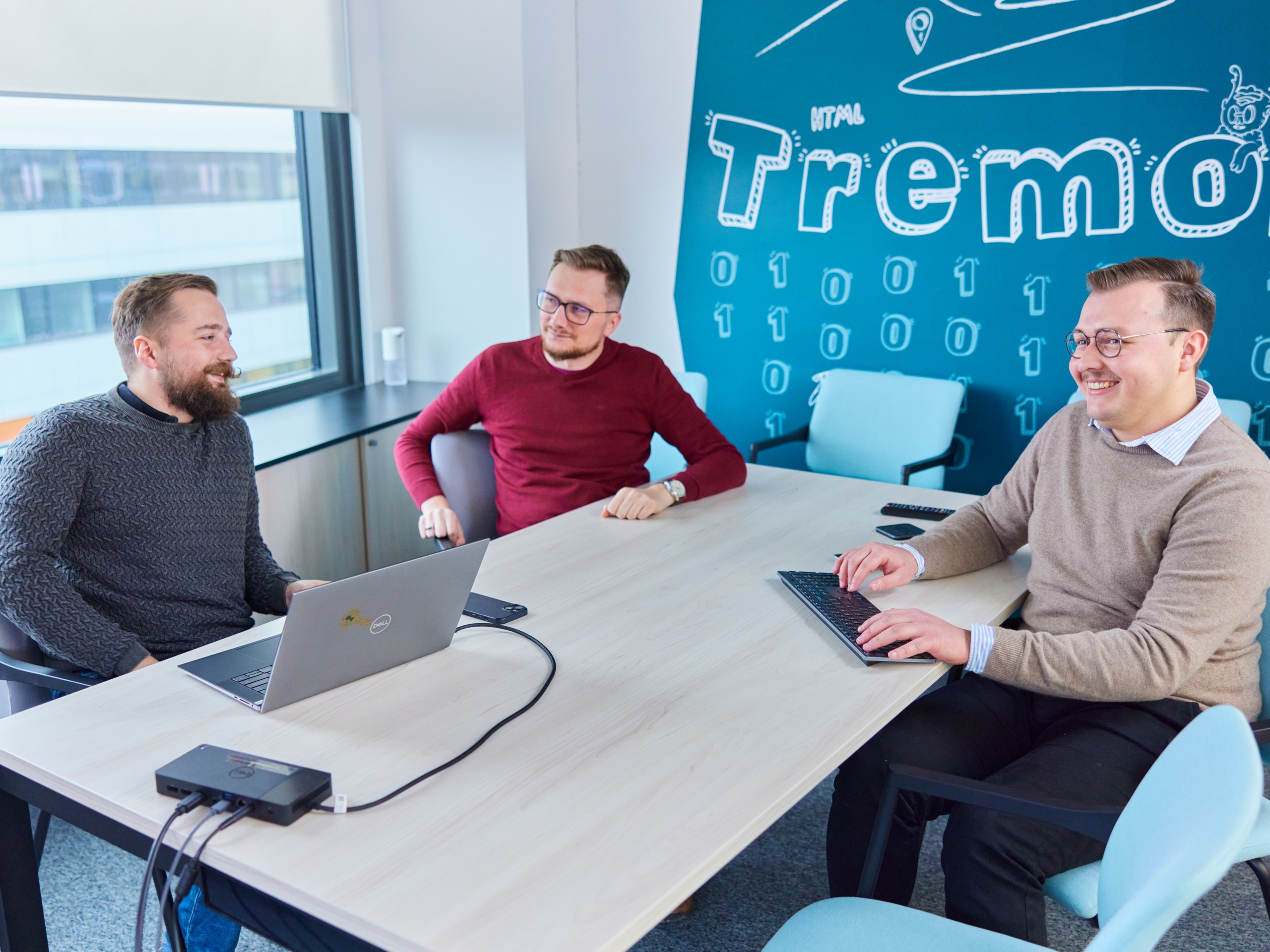
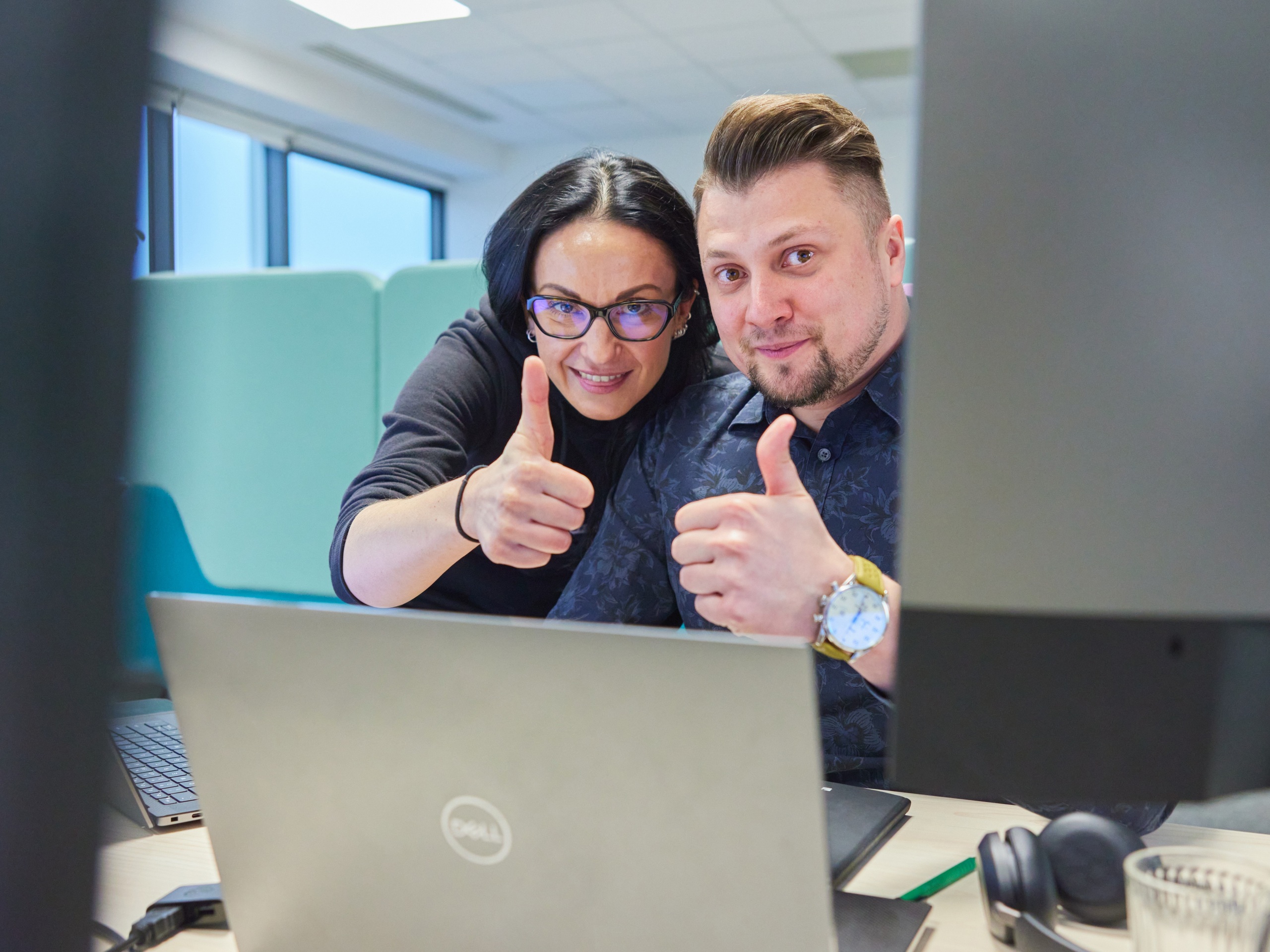
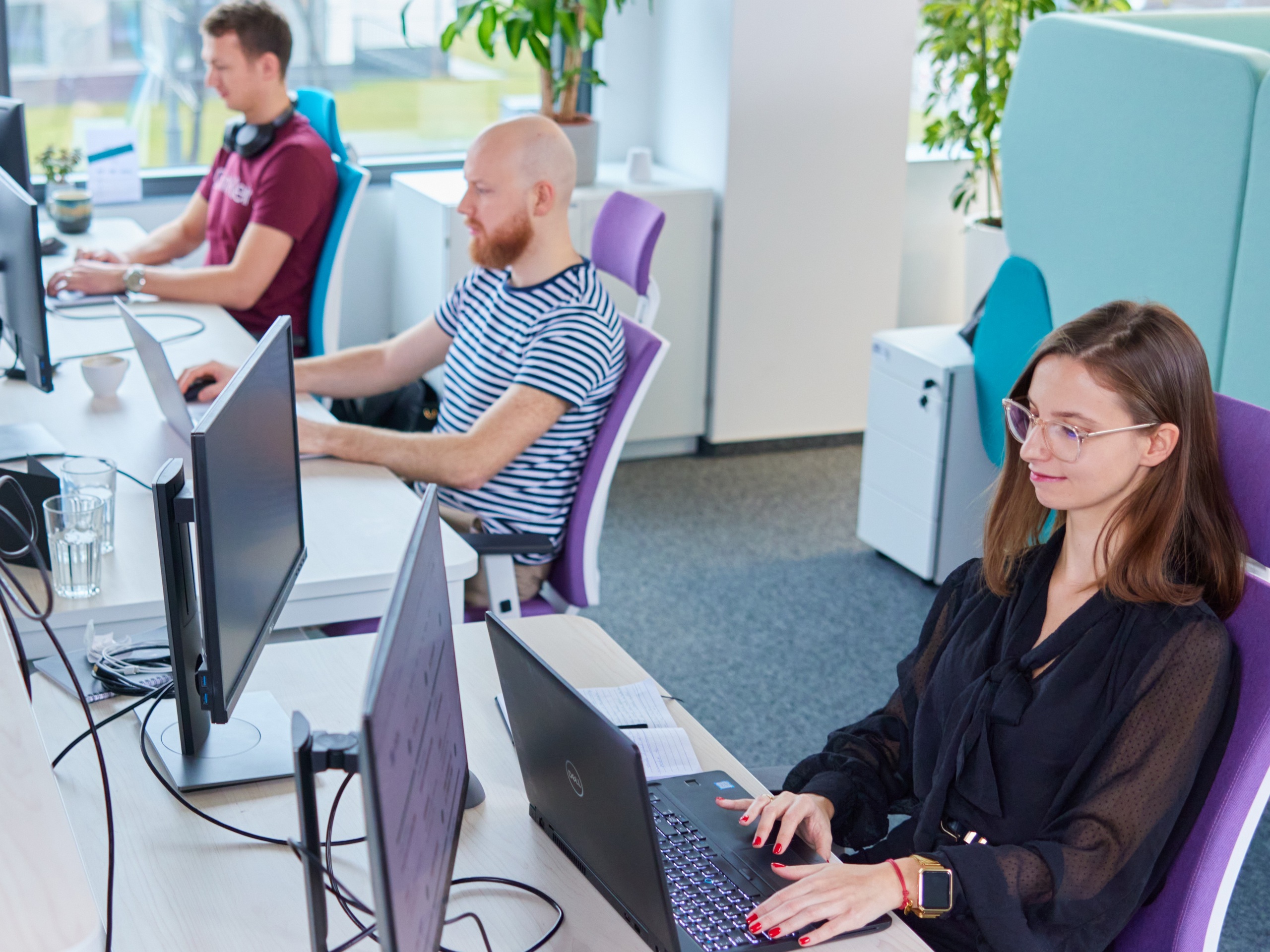
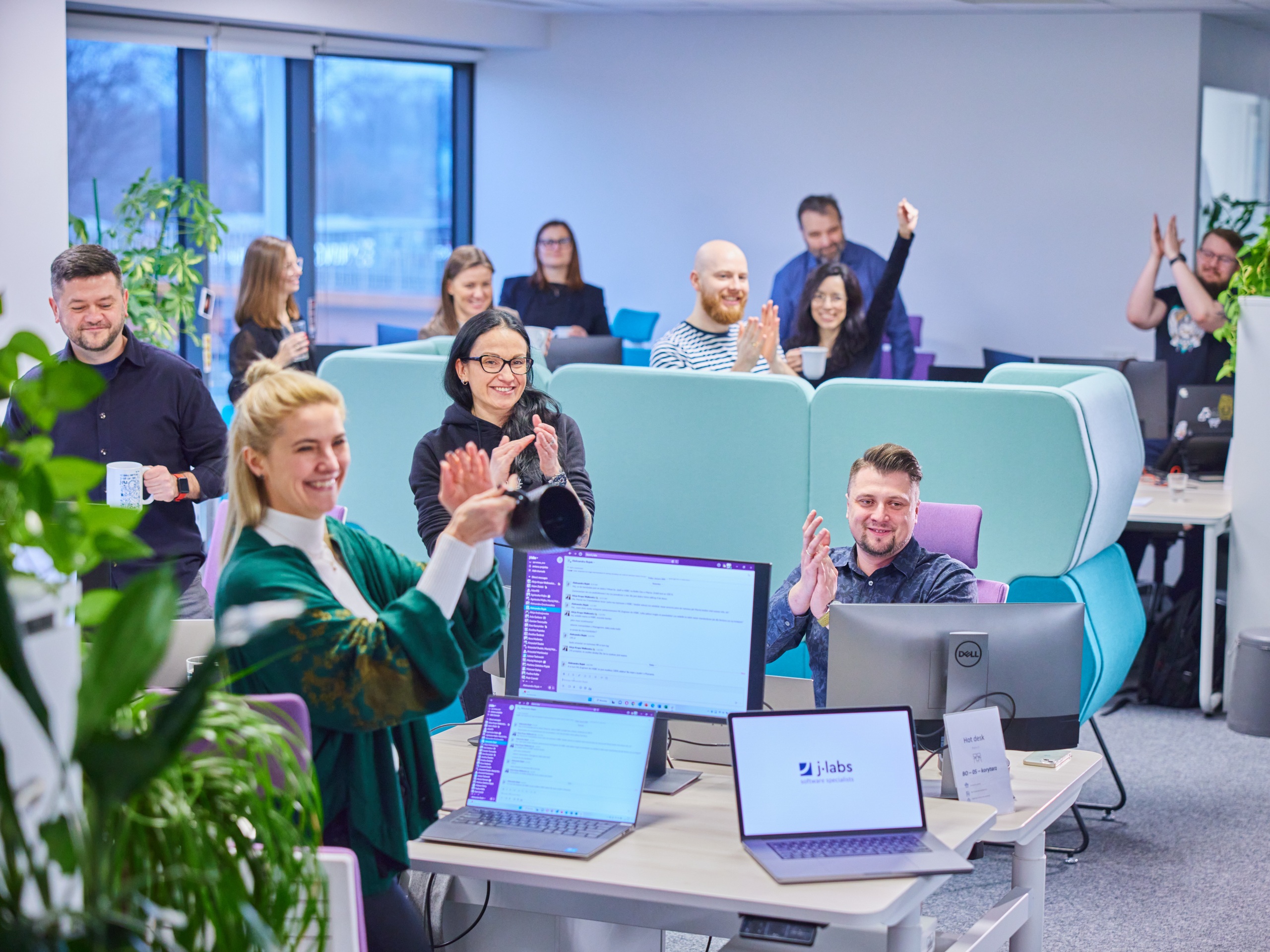