How to use the useEffect hook with the AbortController
In modern web development, handling asynchronous operations, such as fetching data from APIs, is a common requirement. However, sometimes these operations may take longer than expected, leading to performance issues and unnecessary resource consumption. To resolve this, JavaScript introduced the AbortController
interface, which provides a way to cancel ongoing asynchronous operations. When combined with React’s useEffect hook, AbortController
allows us to effectively manage asynchronous requests and prevent memory leaks. In this article I will show you how to use AbortController
with useEffect
in React.
What is the AbortController?
The AbortController
interface is built into modern browsers and provides a simple mechanism to abort asynchronous tasks. It consists of an AbortController
object and an associated AbortSignal
object. The AbortController
exposes the abort()
method to cancel an ongoing operation, while the AbortSignal
object has an aborted property indicating whether the operation was aborted.
Why should we use it?
Using AbortController when making API requests provides several benefits:
- Efficient Resource Management: In scenarios where an API request takes longer to complete, canceling the request with
AbortController
prevents unnecessary resource consumption. By aborting the request, you release network resources, memory, and processing power associated with the ongoing operation, thereby improving overall performance. - Faster User Experience: If a user initiates multiple API requests and quickly navigates away from a page or performs another action, canceling the pending requests using
AbortController
ensures that the application doesn’t waste time and resources processing responses that are no longer needed. This leads to a faster and more responsive user experience. - Preventing Race Conditions: In some cases, multiple API requests can be initiated concurrently. With
AbortController
, you can cancel previous requests when new ones are triggered, preventing race conditions where outdated responses overwrite the results of the most recent request. This ensures that the displayed data remains accurate and up-to-date. - Neat Error Handling: When you cancel an API request using
AbortController
, the associated promise is rejected with anAbortError
. This allows you to handle cancellation as a specific error case and implement appropriate error handling logic. It provides better control over how your application responds to canceled requests, enabling you to display informative messages or perform fallback actions. - Scalability and Performance Optimization: For complex applications with numerous components and data-fetching operations, managing ongoing API requests becomes crucial for scalability and performance. By using
AbortController
, you can effectively handle request cancellation and cleanup, preventing memory leaks and unnecessary resource usage, thereby improving the scalability and performance of your application.
How to use it in Javascript?
Here is a basic example of how you can use AbortController
to abort a fetch request:
const controller = new AbortController();
const signal = controller.signal;
/// Start the fetch request
fetch('https://api.example.com/data', { signal })
.then(response => {
// Process the response
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Request aborted');
} else {
console.log('Error:', error);
}
});
// Abort the request after 5 seconds
setTimeout(() => {
controller.abort();
}, 5000);
The AbortController
interface consists of two main parts: the AbortController
object and the AbortSignal
object.
AbortController
: This is the main object that controls the abortion of an asynchronous operation. It has two methods: –abort()
: This method aborts the associated operation by canceling any pending requests or actions. It raises an AbortSignal event, indicating that the operation was aborted. –signal
: This property returns anAbortSignal
object associated with the controller.AbortSignal
: This is an object that represents the signal used for cancellation. It has a single property: –aborted
: This property is a Boolean that indicates whether the associated operation has been aborted (true
) or not (false
).
How to use it in React with a useEffect
hook?
The useEffect
hook in React allows us to perform side effects, such as fetching data, when the component mounts, updates, or unmounts. By integrating AbortController
with useEffect
, we can cancel ongoing requests when the component unmounts or when a dependency changes.
Here’s an example of how to use AbortController
with useEffect
:
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
const controller = new AbortController();
const signal = controller.signal;
// Start the asynchronous operation
fetchData(signal)
.then(response => {
// Handle the response
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Request aborted');
} else {
console.log('Error:', error);
}
});
return () => {
// Abort the request when the component unmounts or when a dependency changes
controller.abort();
};
}, []);
return <div>My Component</div>;
};
export default MyComponent;
In the example above, we have created an instance of AbortController
and obtained the associated AbortSignal
within the useEffect
hook. We have initiated the asynchronous operation, fetchData()
, passing the signal as an argument. If the component unmounts or if a dependency changes, the cleanup function returned by useEffect is triggered. Inside the cleanup function, we have called abort()
on the controller to cancel the request.
By integrating AbortController
with useEffect
, we ensure that any ongoing asynchronous operation is properly canceled when it is no longer needed. This prevents potential memory leaks and improves the overall performance of our React application.
Final words
Asynchronous operations are an essential part of web development, but it’s crucial to manage them effectively. With the AbortController
interface and the useEffect
hook in React, we can easily handle asynchronous requests and prevent unnecessary resource consumption. By canceling ongoing operations when components unmount or when dependencies change, we can improve performance and ensure a smoother user experience. The combination of AbortController
and useEffect
is a powerful tool in our toolbox for managing asynchronous tasks in React applications.
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us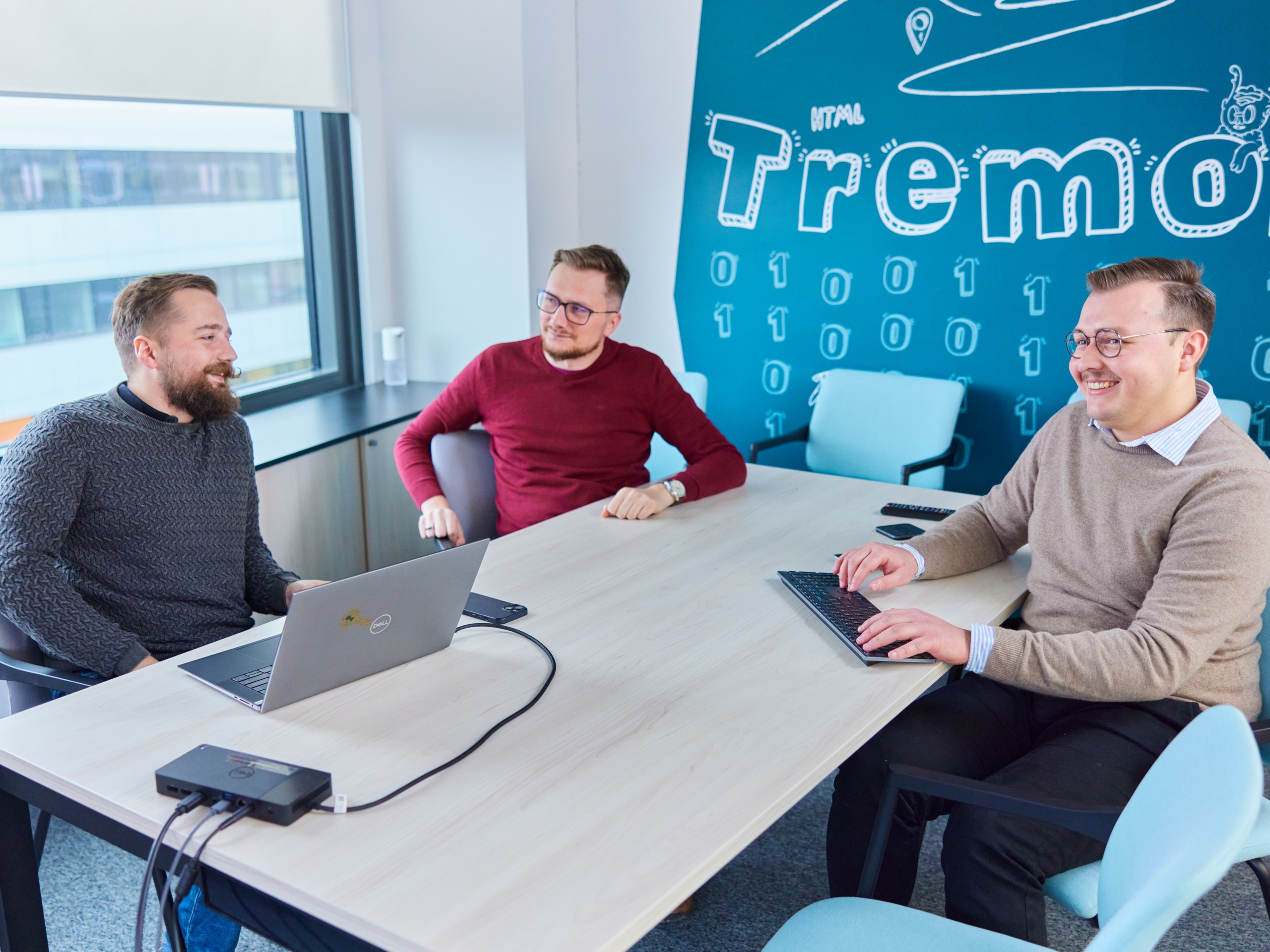
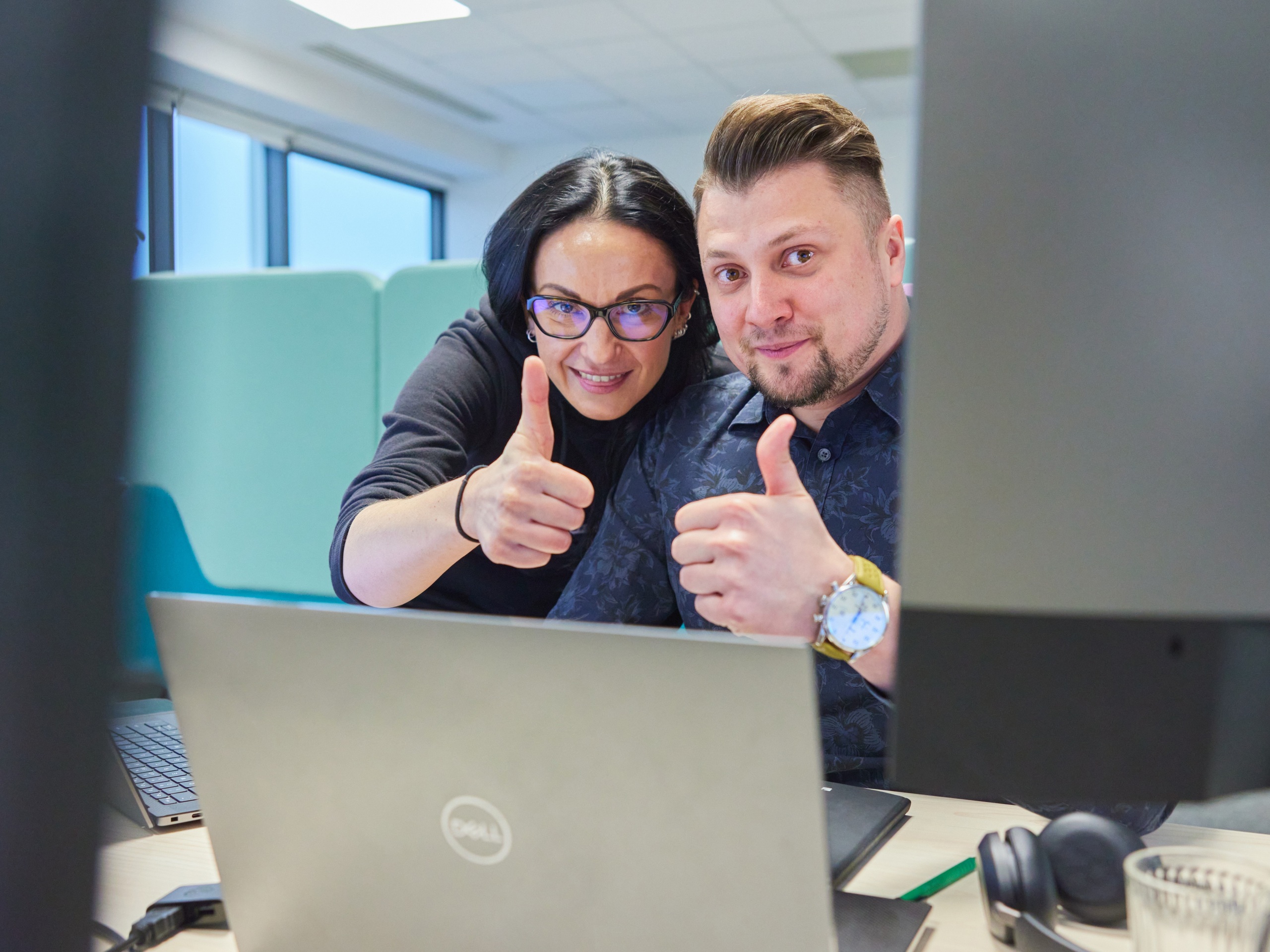
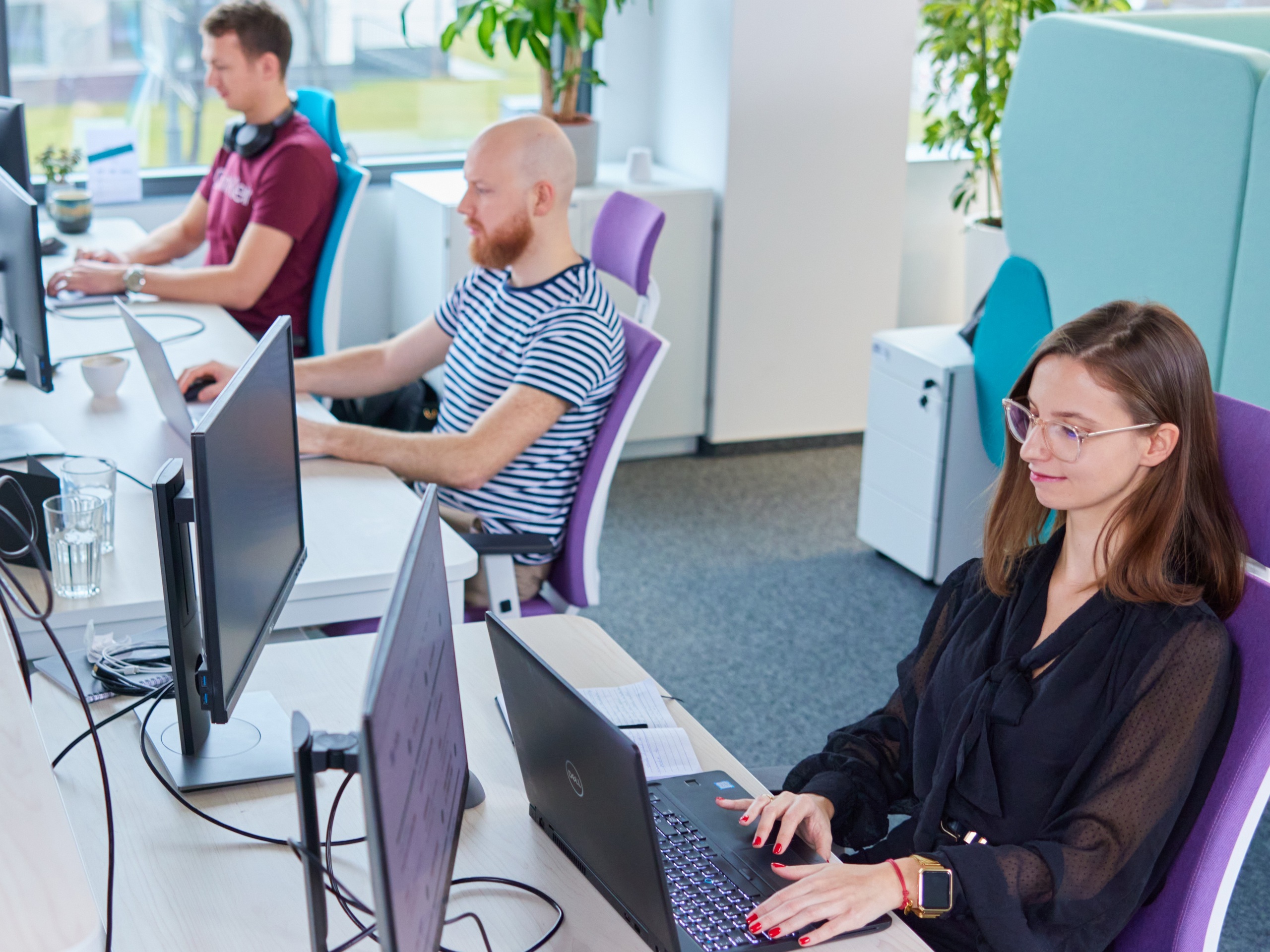
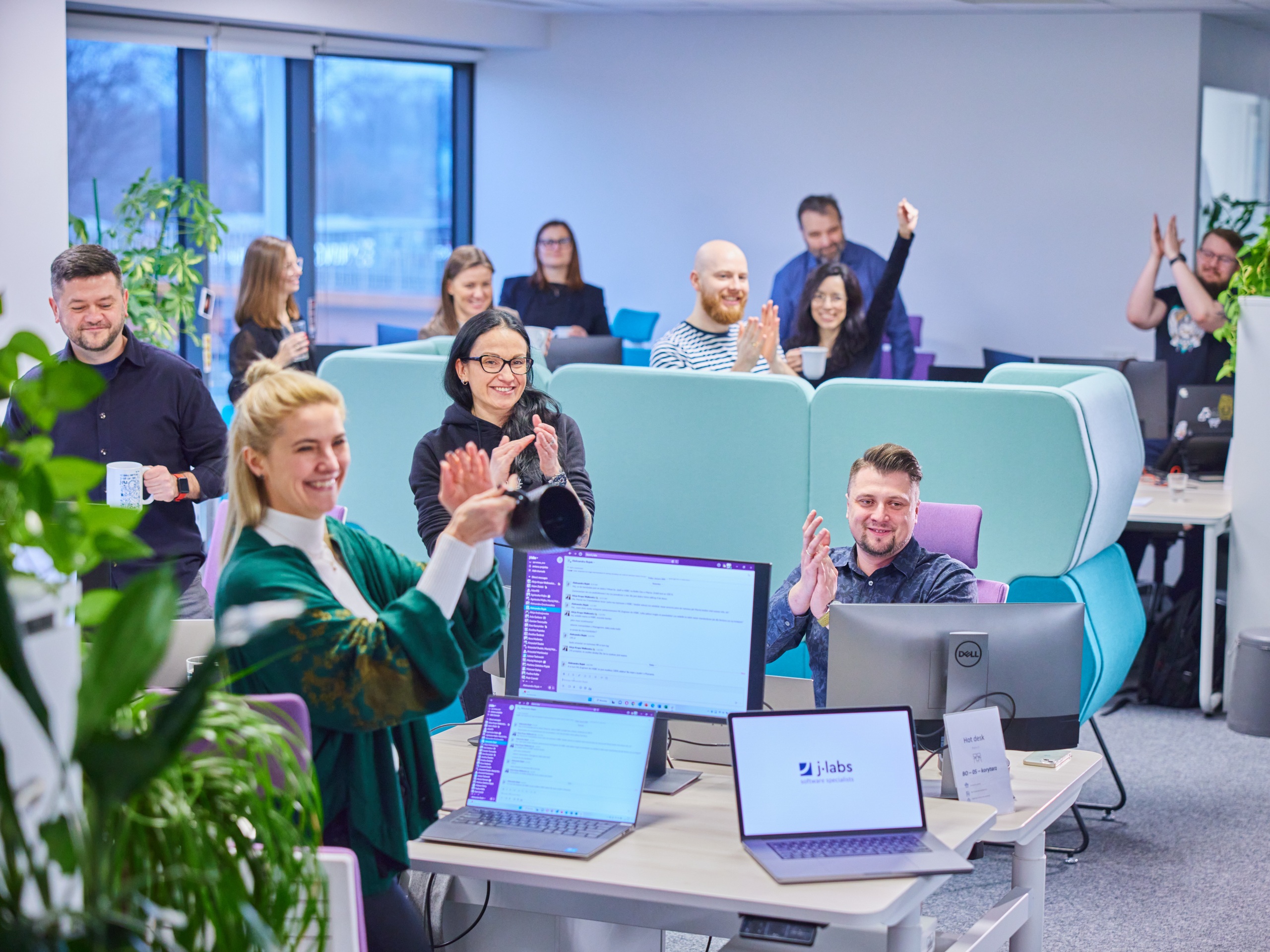