JSON Web Token handling with Rest Assured
Introduction to JWT
JWT, which stands for JSON Web Token, is a compact and self-contained way of securely transmitting information between two parties. It is often used for authentication and authorization purposes in web applications, APIs, and distributed systems. In simple terms, JWT is like a digital passport or an ID card that contains encoded information about a user or an entity. It consists of three parts: a header, a payload, and a signature. The header specifies the type of token (which is JWT) and the algorithm used to sign it. The payload contains the actual data or claims, which can include information such as the user’s ID, role, permissions, and other relevant details. These claims are encoded as a JSON object. To ensure the integrity and authenticity of the token, a signature is generated by combining the header, payload, and a secret key known only to the server. This signature is added to the token, making it tamper-proof. When the token is received by the server, it can verify the signature using the same secret key and ensure that the token has not been modified or tampered with.
Example:
Header:
{
"alg": "HS256",
"typ": "JWT"
}
Payload:
{
"name": "admin",
"age": 35
}
Signature:
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
my_secret
)
Encoded:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJuYW1lIjoiYWRtaW4iLCJhZ2UiOjM1fQ.zt3sQAm2-9gjbRSh20rjn1nAPIv3vNhgQM2-31I5DRY
How to simply test JWT with the RestAssured framework
For my simple test case I’m going to use the https://demoqa.com/ web page, which was intended to be tested.
First of all we have to setup a few things. According to the DemoQA swagger, I should create a user by sending POST
to the /Account/v1/User
endpoint with the user data:
private String body = "{\n" +
" \"userName\": \"" + USERNAME + "\",\n" +
" \"password\": \"" + PASSWORD + "\"\n" +
"}";
Post the user and retrieve its ID in response:
private void createUser() {
userID = given()
.contentType("application/json")
.body(body)
.when()
.post(USER_ENDPOINT)
.jsonPath()
.get("userID");
}
When the user is successfully created it is time to generate the token for him. Following the swagger, we have to send POST
with the same user data to /Account/v1/GenerateToken
endpoint:
private void generateToken() {
token = given()
.contentType("application/json")
.body(body)
.when()
.post(GENERATE_TOKEN_ENDPOINT)
.jsonPath()
.get("token");
}
Like in the previous code snippet the token was retrieved from the json response, taking the value of the “token” member.
After this short setup we can perform two simple test cases with sending GET
to /Account/v1/User
. In the first case we are going to authorize the request with the already retrieved token, while in the second one not.
GET the authorized user:
@Test
void getAuthorizedUser() {
given()
.contentType("application/json")
.headers("Authorization", "Bearer " + token)
.when()
.get(USER_ENDPOINT + "/" + userID)
.then()
.assertThat()
.statusCode(HttpStatus.SC_OK);
}
GET the unauthorized user:
@Test
void getUnauthorizedUser() {
given()
.contentType("application/json")
.when()
.get(USER_ENDPOINT + "/" + userID)
.then()
.assertThat()
.statusCode(HttpStatus.SC_UNAUTHORIZED);
}
The test cases above are very similar. The only difference is adding the Authorization header with the token in one of the requests. The Bearer schema is a common convention when handling the JWT token in the authorization header:
Authorization: Bearer /token/
As we can see, the response for the authorized user request has the 200 HTTP status code (OK). In the second case, when the user is unauthorized (no token set in the header), the response status code is 401 (unauthorized).
Summary
The article provides a basic guide of handling JSON Web Tokens (JWTs) using the Rest Assured library. The significance of JWTs in securing web applications and APIs is explained. The main part of the article is about the step-by-step process of implementing JWT authentication in Rest Assured. It covers essential concepts such as obtaining and parsing JWTs, setting headers and handling authorized requests. By reading this article you get basic knowledge and tools to successfully implement and validate JWT authentication in your Rest Assured test scenarios, ensuring reliable API testing practices.
Reference
Meet the geek-tastic people, and allow us to amaze you with what it's like to work with j‑labs!
Contact us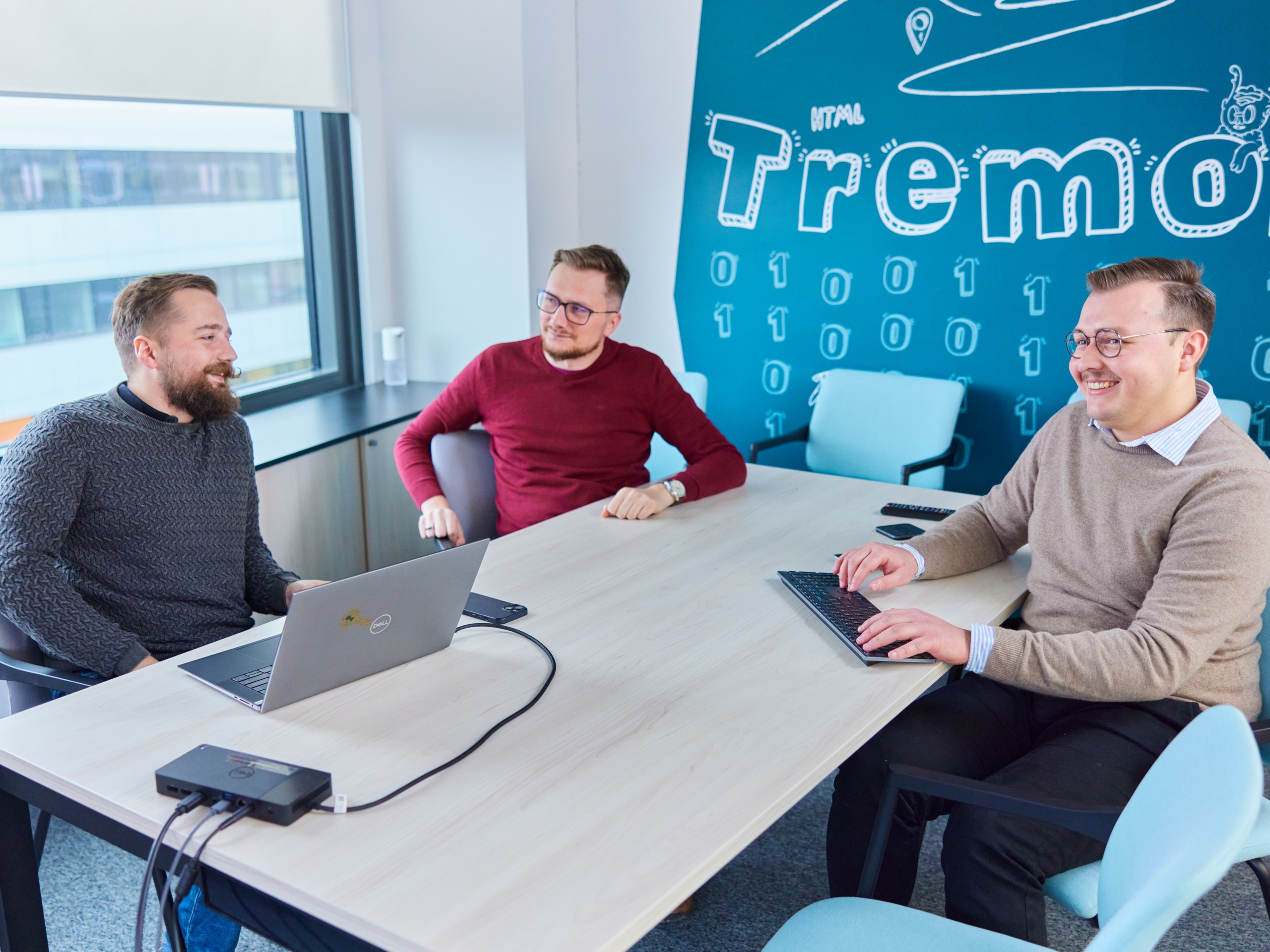
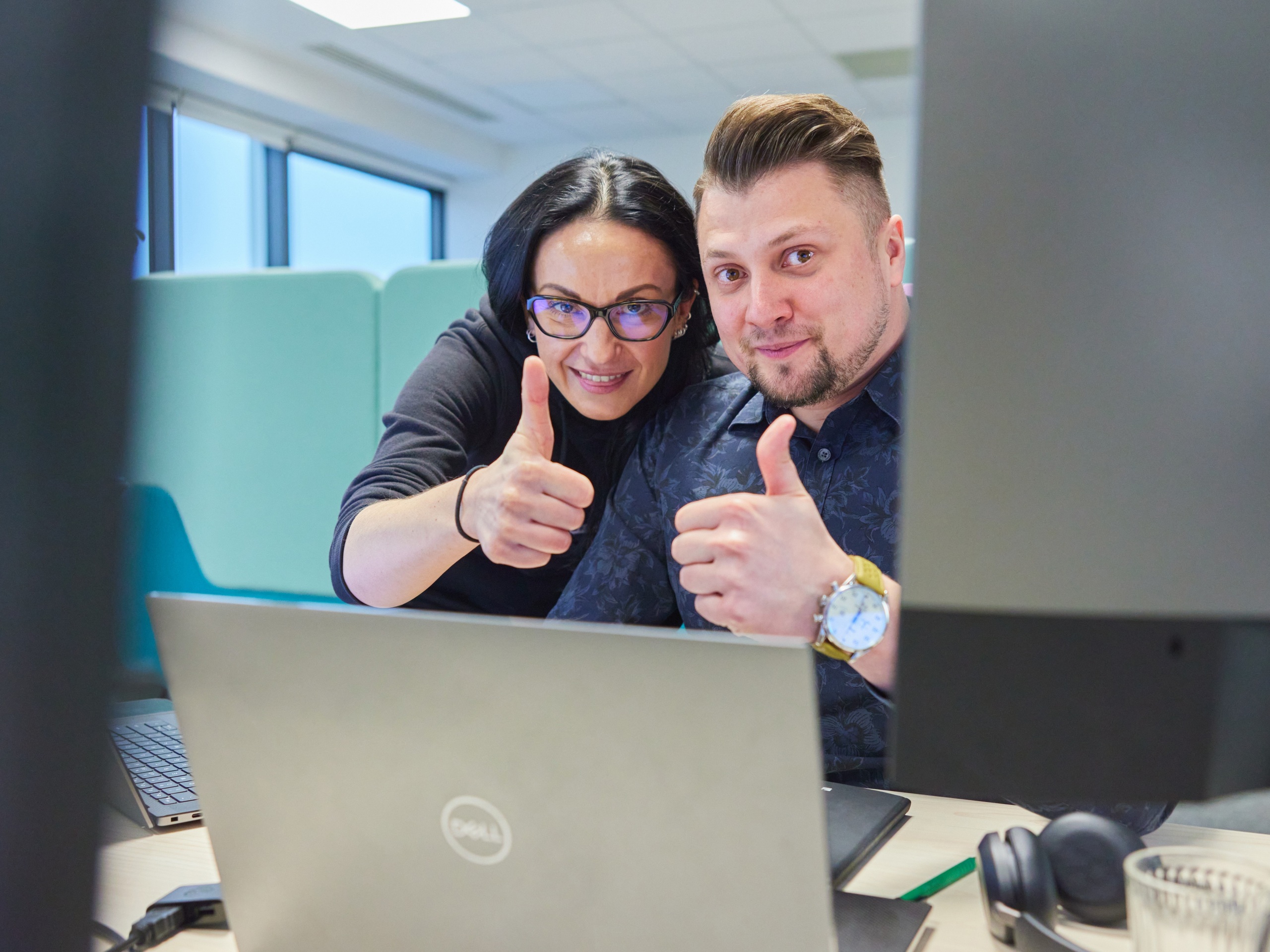
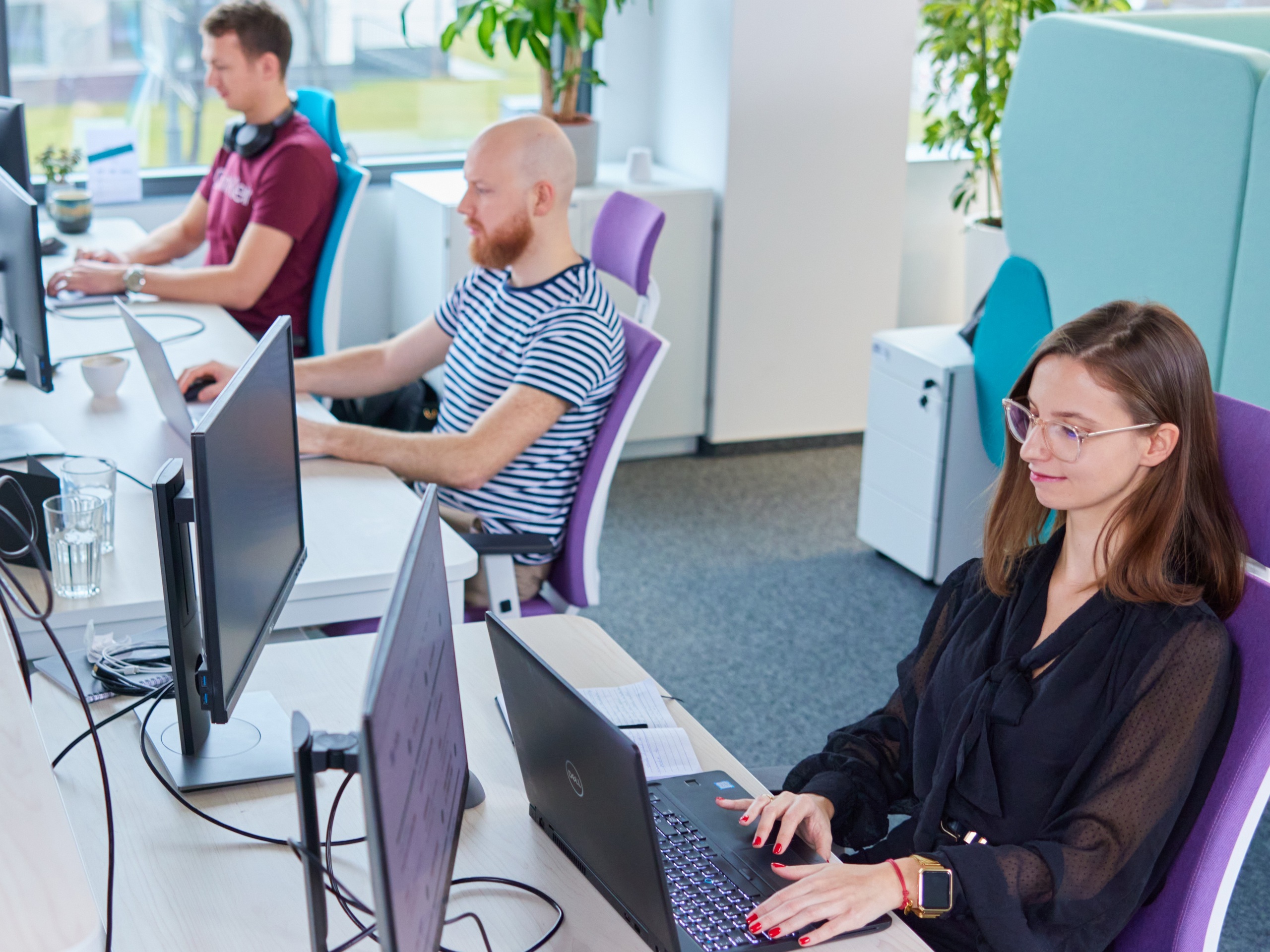
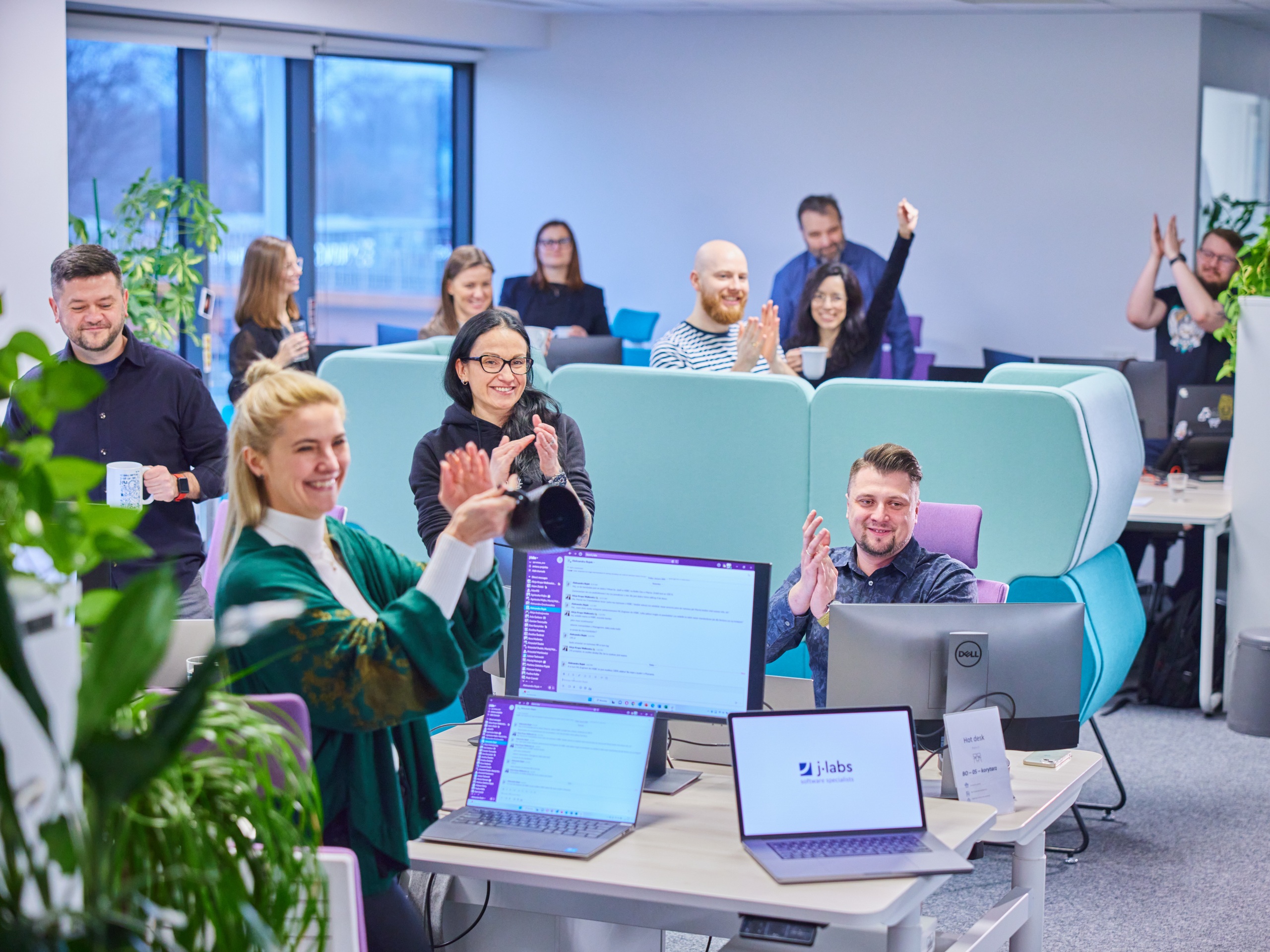