Najnowsze artykuły
Wszystkie artykuły
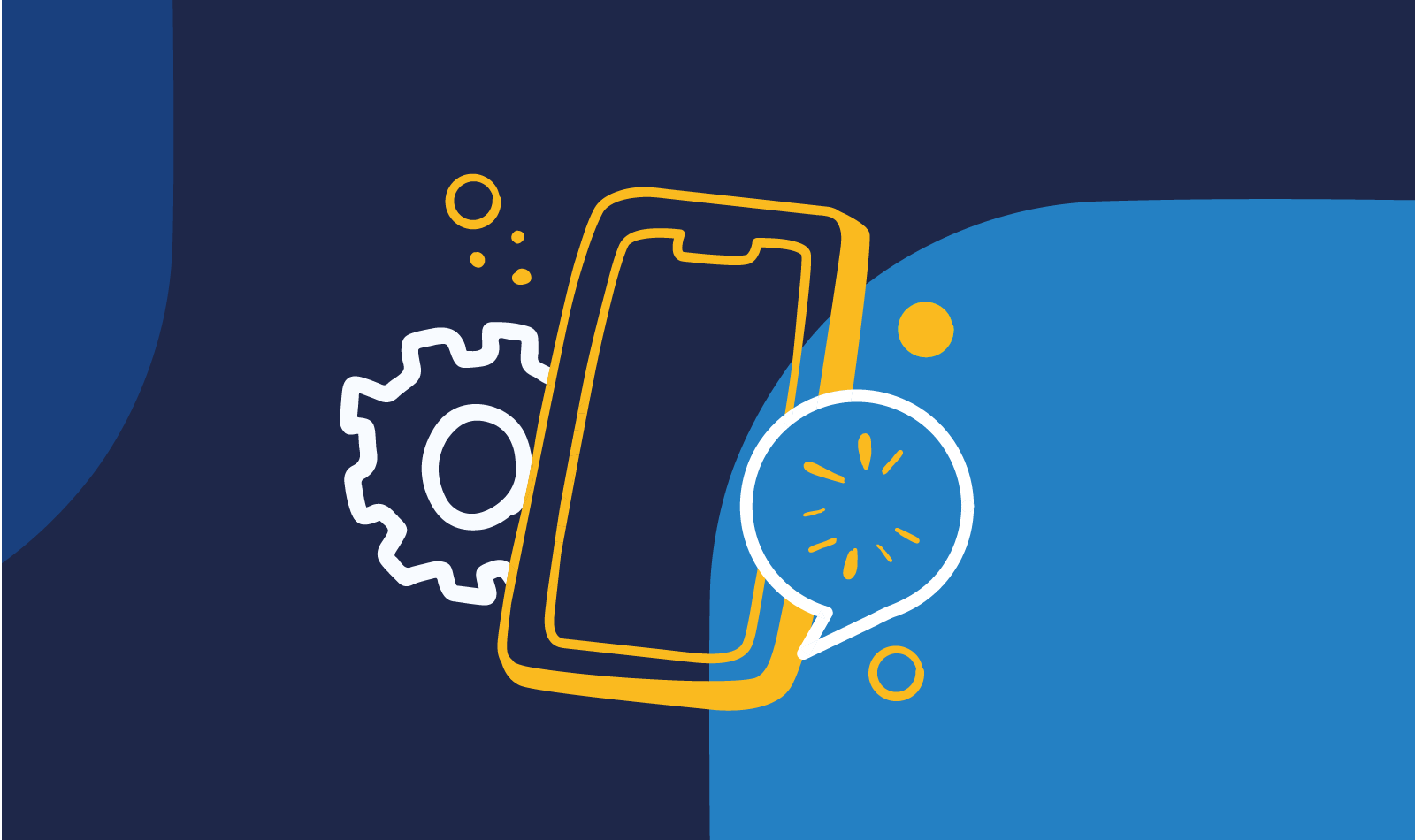
Liquibase: Narzędzie do zarządzania bazami danych
Liquibase to narzędzie do zarządzania bazami danych, które umożliwia zautomatyzowane wprowadzanie zmian, oferując elastyczność i wsparcie dla wielu systemów baz danych. Artykuł opisuje prosty przypadek użycia Liquibase do cofnięcia wprowadzanych zmian, a także pokazuje integrację ze Spring Boot.
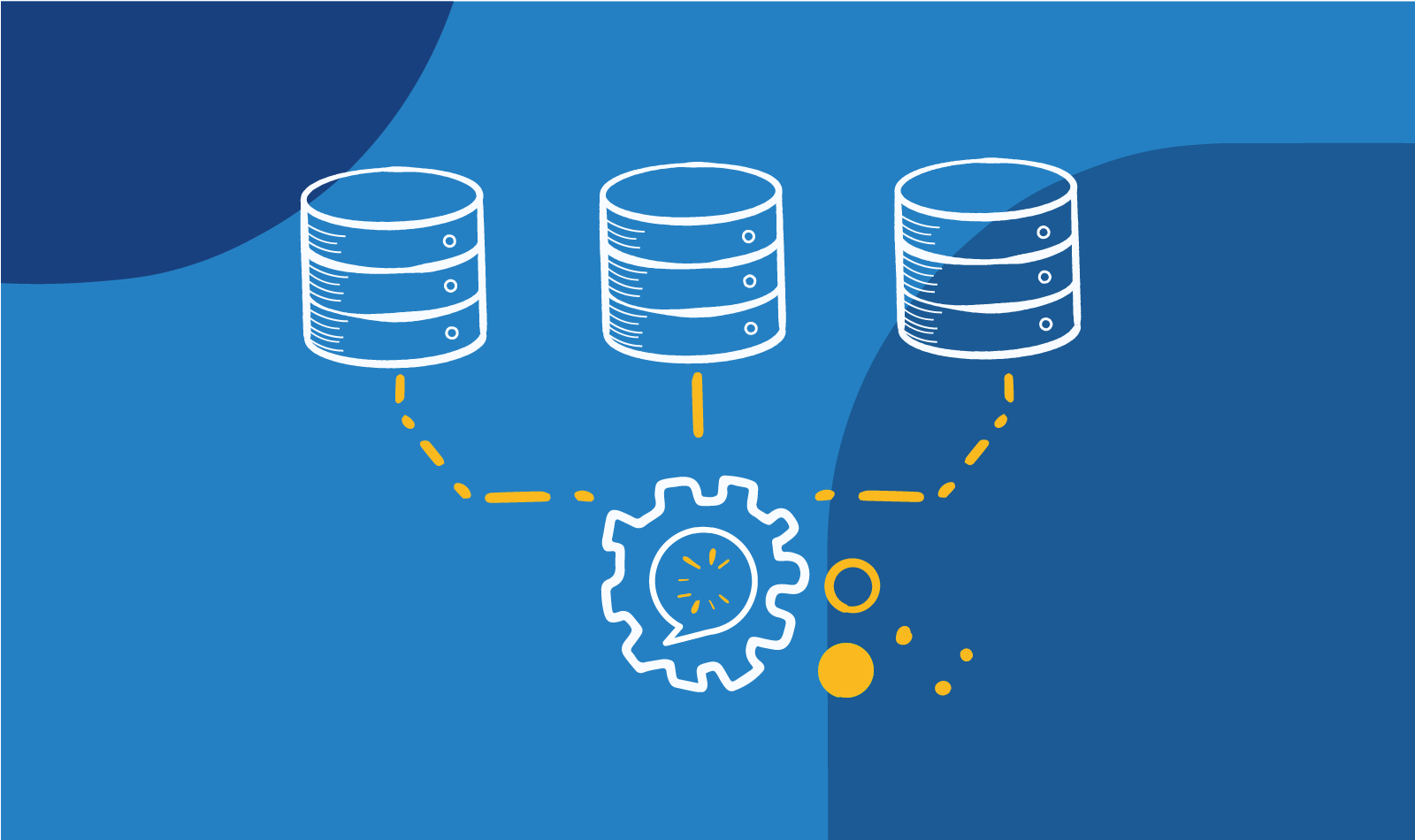
Prowadzenie automatyzacji testów przy użyciu Appium i Cucumber na infrastrukturze opartej na chmurze
W tym artykule zagłębiamy się w detale związane z automatyzacją testów przy użyciu dynamicznej kombinacji Appium i Cucumber na platformie chmurowej - HeadSpin.
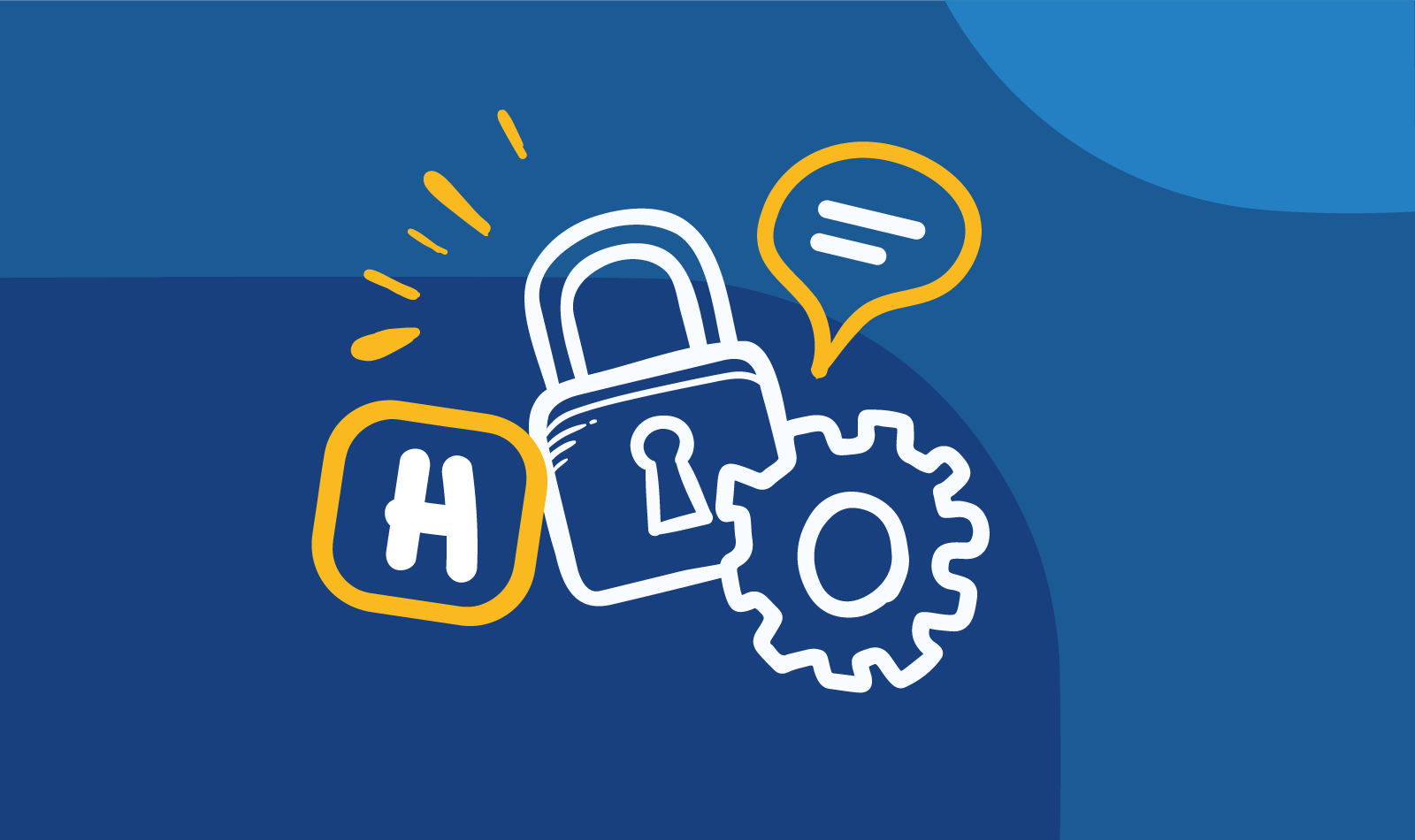
Rozproszona blokada przy użyciu Hazelcast’a
Synchronizowanie dostępu do zasobów współdzielonych jest głównym problemem aplikacji wielowątkowych. Jeśli w celu poprawy wydajności dołożymy kolejne maszyny - sytuacja komplikuję się jeszcze bardziej. Czy synchronizacja systemu rozproszonego jest możliwa beż używania skomplikowanych rozwiązań? W tym artykule chciałbym przedstawić implementację rozproszonych blokad (ang. distributed lock), której byłem częścią.
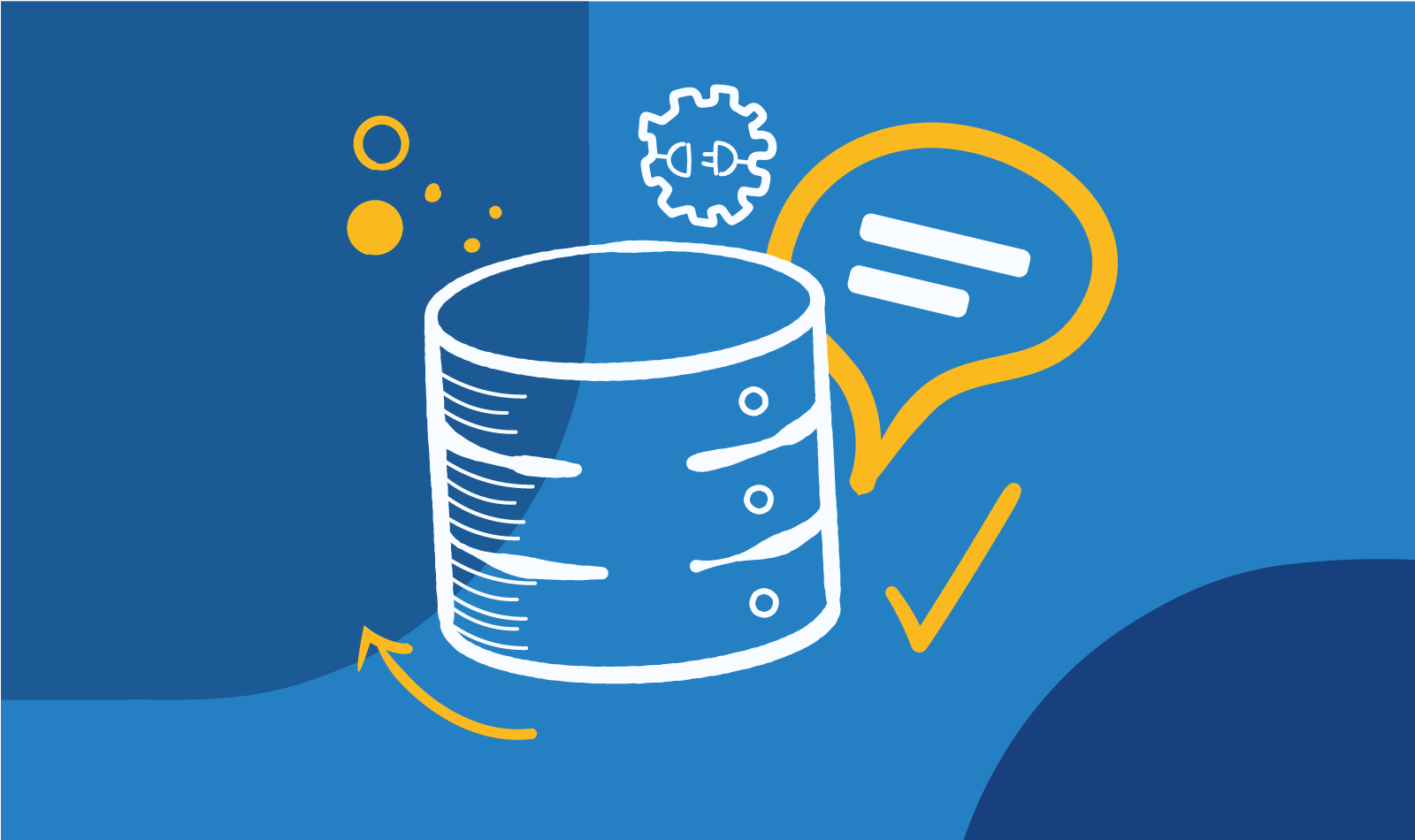
Soap vs Rest
Komunikacja poprzez protokół SOAP oraz porównanie jej z komunikacją w architekturze REST
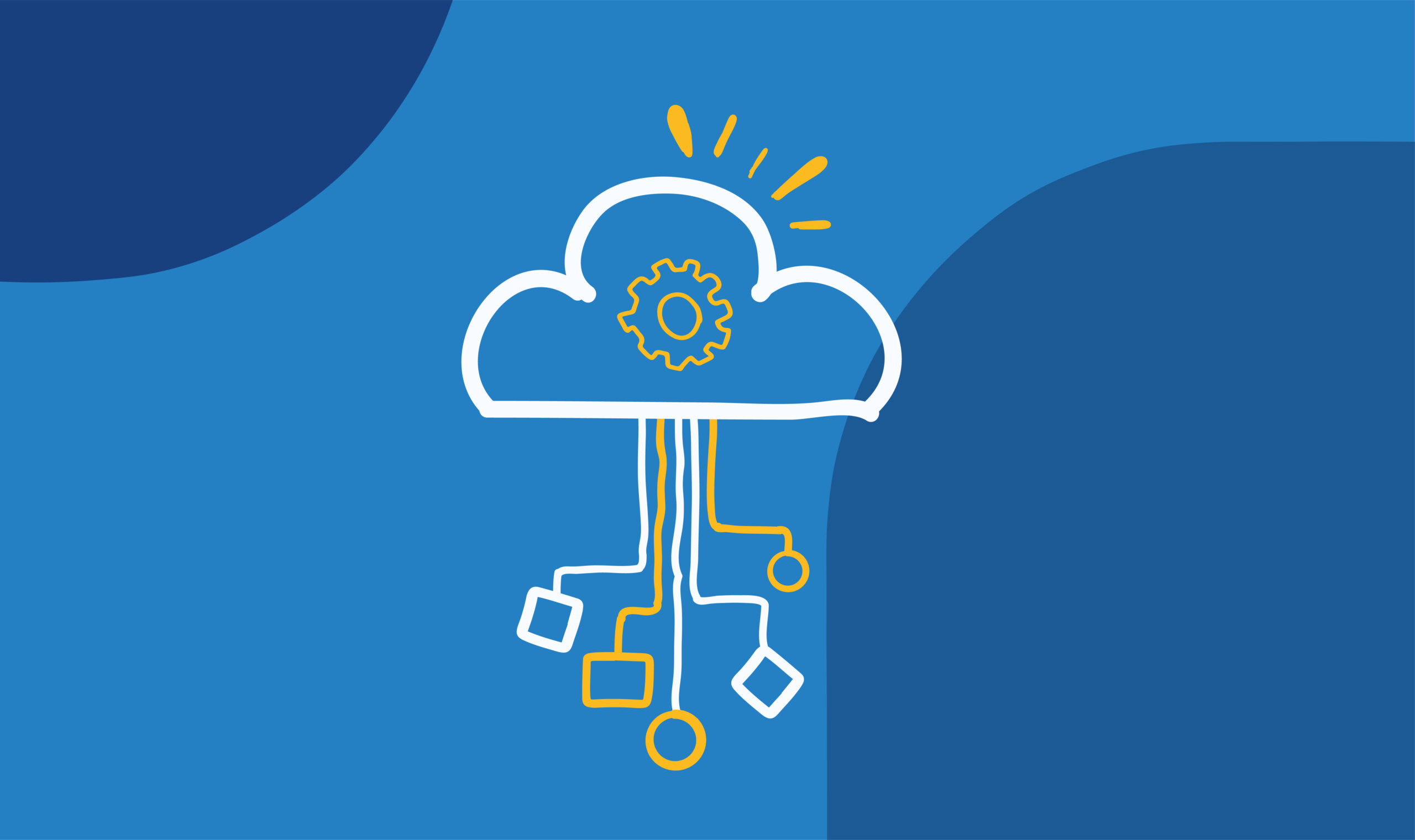
AWS EKS Auto Mode
Wprowadzenie Re:Invent 2024 należy już do historii. I to jakiej historii, mówię wam! Amazon ogłosił dziesiątki nowych funkcji dla AWS Cloud, wiele z nich jest […]
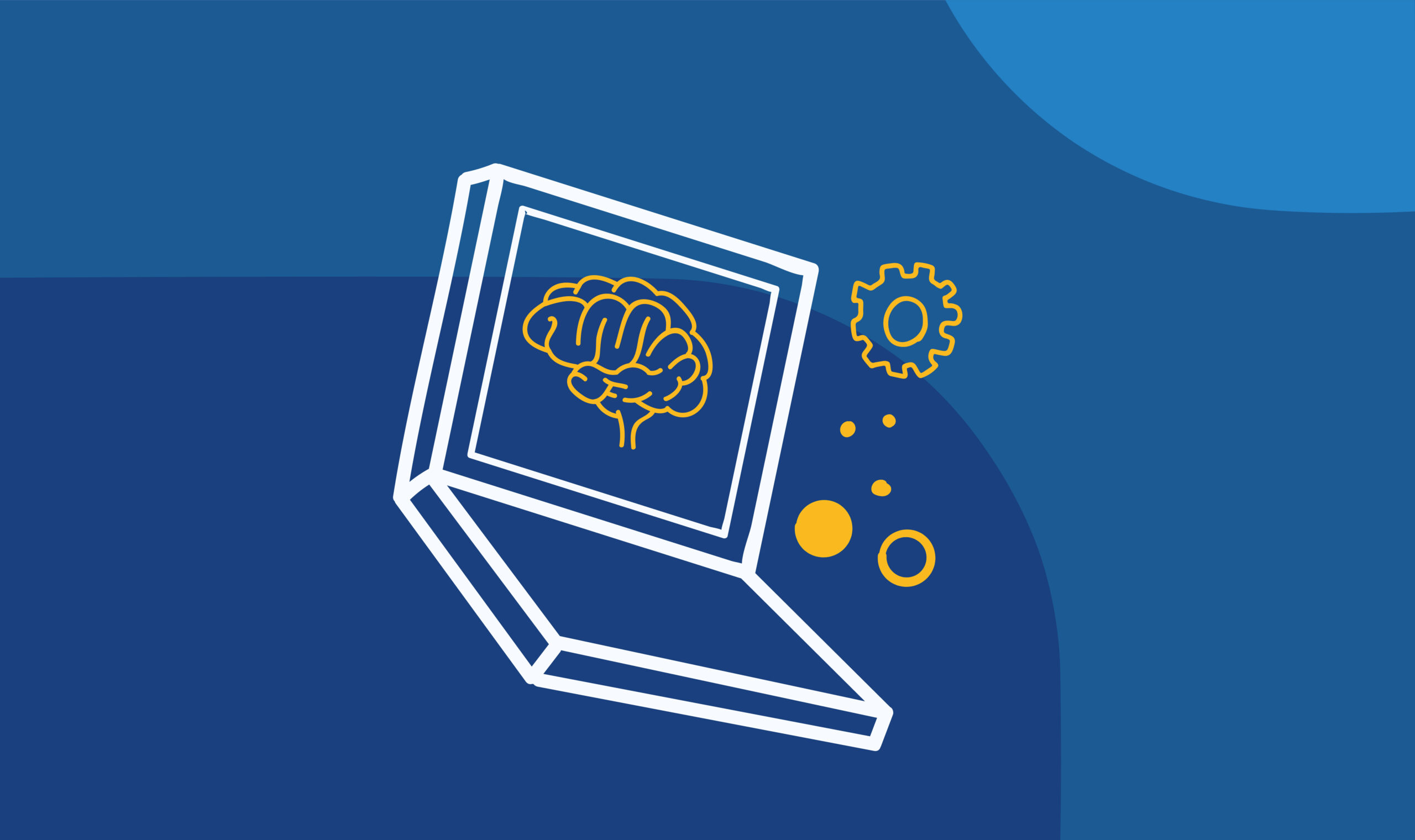
Narzędzia AI ułatwiające pisanie kodu
Współcześnie obserwujemy znaczący rozwój sztucznej inteligencji. Ma ona wpływ m.in. na pracę developerów. Czy AI będzie w stanie kompletnie zastąpić programistów? Raczej nie ma na […]
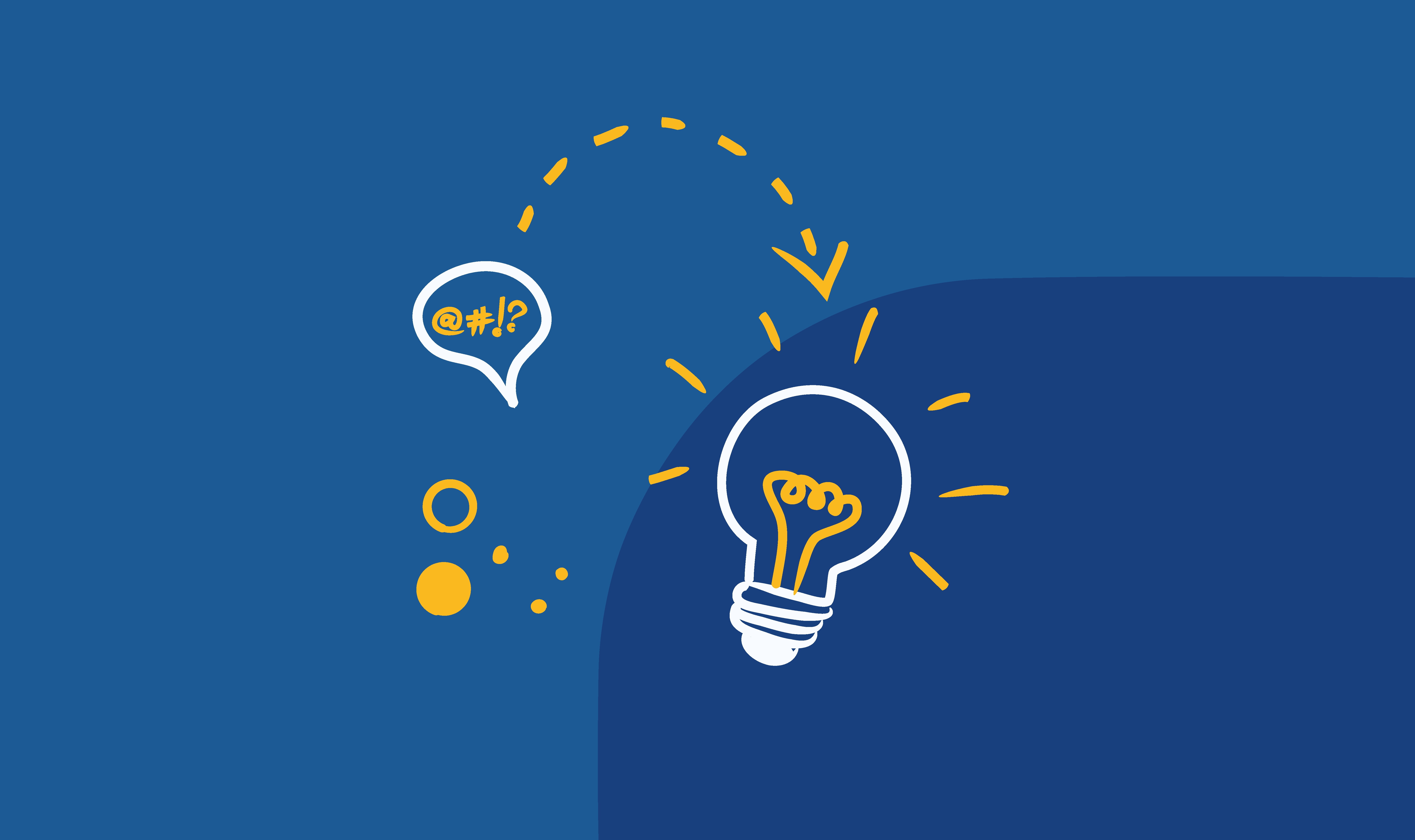
Nawigacja po algorytmach numerycznych za pomocą języka Java
Nawigacja po algorytmach numerycznych za pomocą języka Java: podróż do rozwiązywania problemów matematycznych.
Artykuł ten zagłębia się w dziedzinę algorytmów numerycznych, dostarczając kompleksowego przewodnika po ich znaczeniu, zasadach i praktycznych implementacjach przy użyciu języka programowania Java.
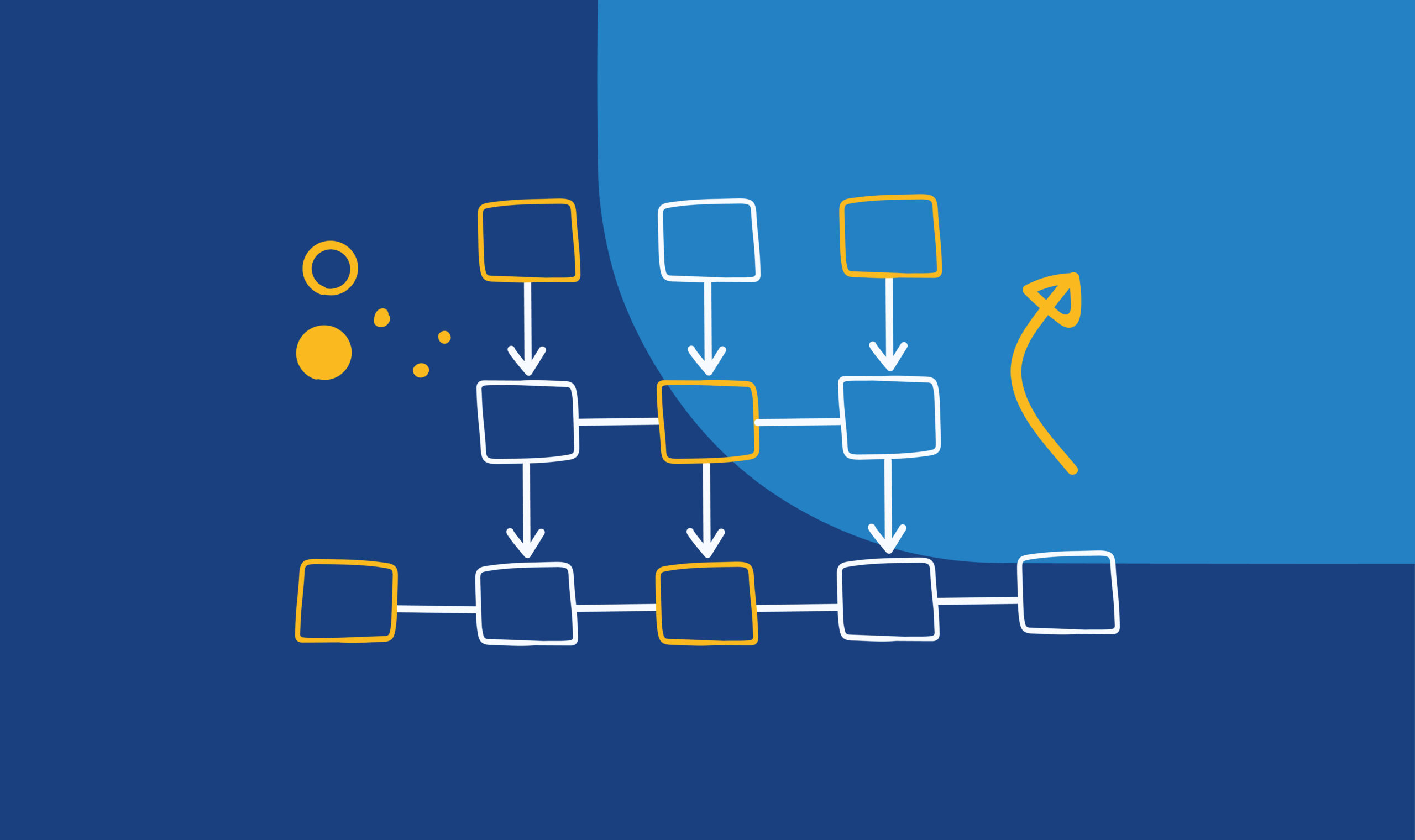
Współbieżność w Javie: Zaawansowane funkcje
W dynamicznym środowisku rozwoju nowoczesnego oprogramowania tworzenie solidnych i responsywnych aplikacji wymaga skutecznego zarządzania współbieżnością. Kamień węgielny stanowi pakiet Java.util.concurrent, oferujący bogaty zestaw narzędzi do obsługi złożonych scenariuszy wielowątkowych.
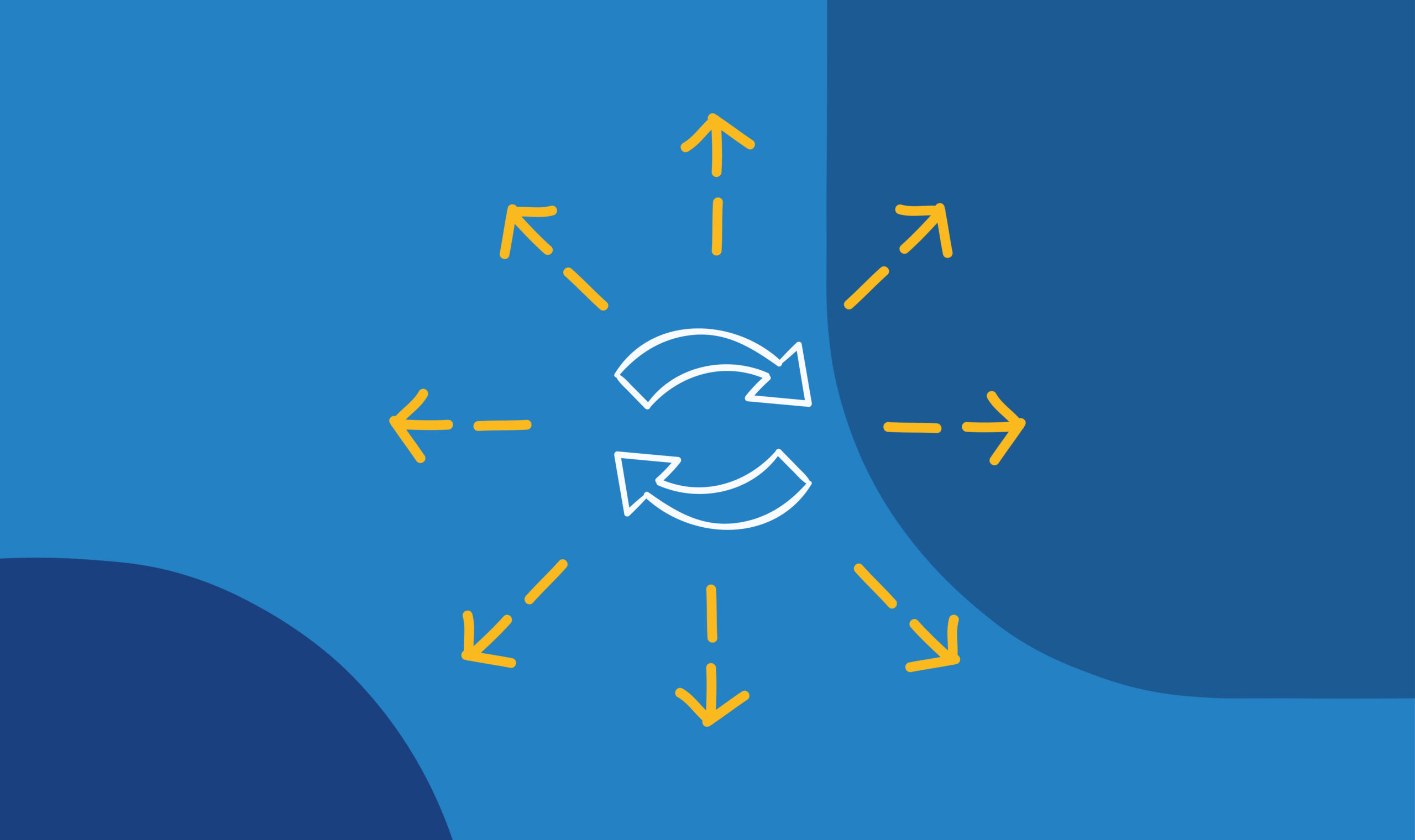
Współbieżność w Javie – synchronizacja i wielowątkowość
Artykuł zagłębia się w tematykę programowania wielowątkowego w Javie, oferując kompleksowe zrozumienie podstaw wielowątkowości, podstawowych technik synchronizacji oraz zawiłości zarządzania współbieżnym wykonaniem.
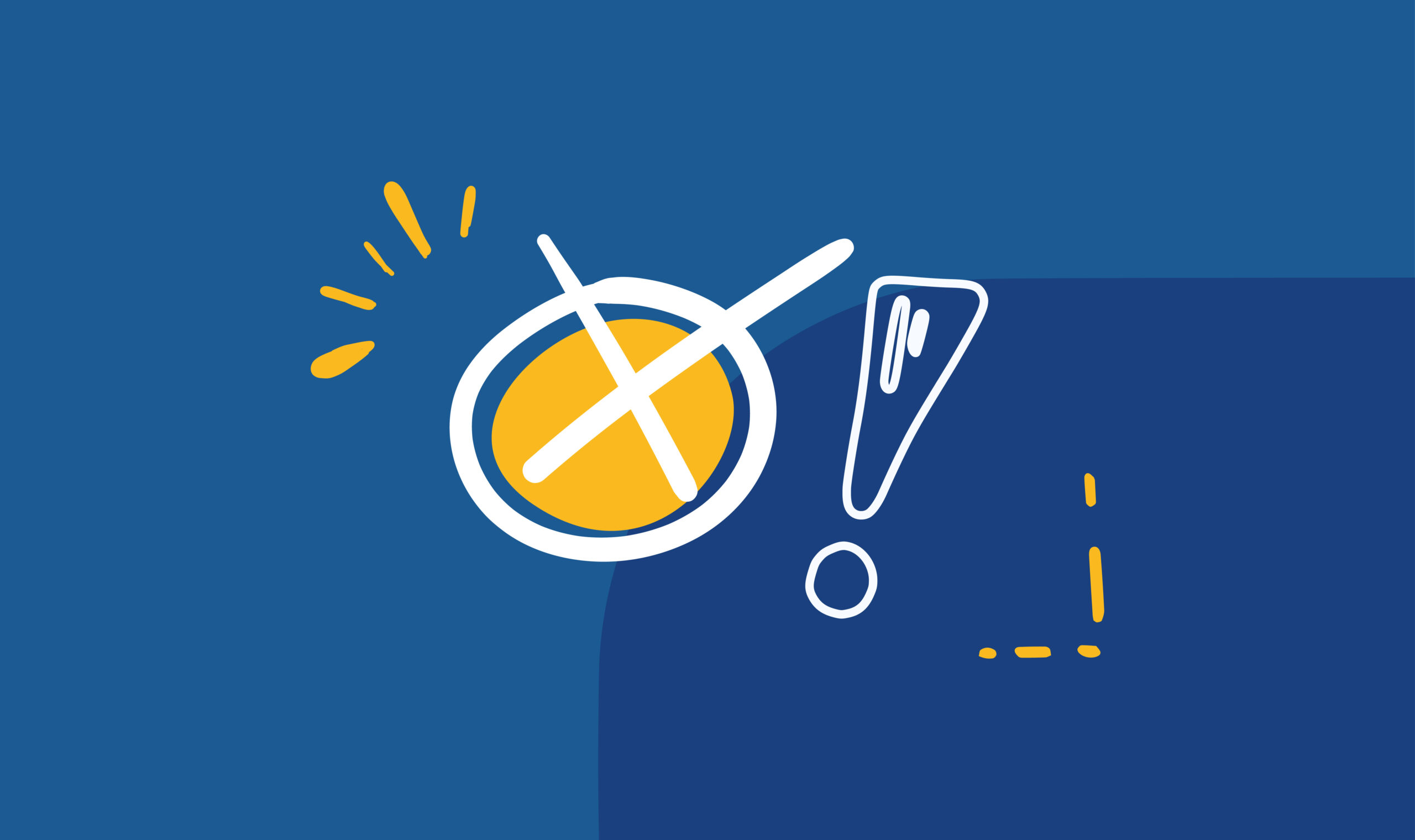
Obsługa wyjątków w Javie – strategie i najlepsze praktyki
Artykuł zagłębia się w sztukę efektywnego zarządzania wyjątkami w aplikacjach Java. Obsługa wyjątków jest krytycznym aspektem pisania solidnego i łatwego w utrzymaniu kodu, dlatego ten artykuł zawiera kompleksową analizę strategii i praktyk, które prowadzą do czystszych i bardziej odpornych programów.
Poznaj mageek of j‑labs i daj się zadziwić, jak może wyglądać praca z j‑People!
Skontaktuj się z nami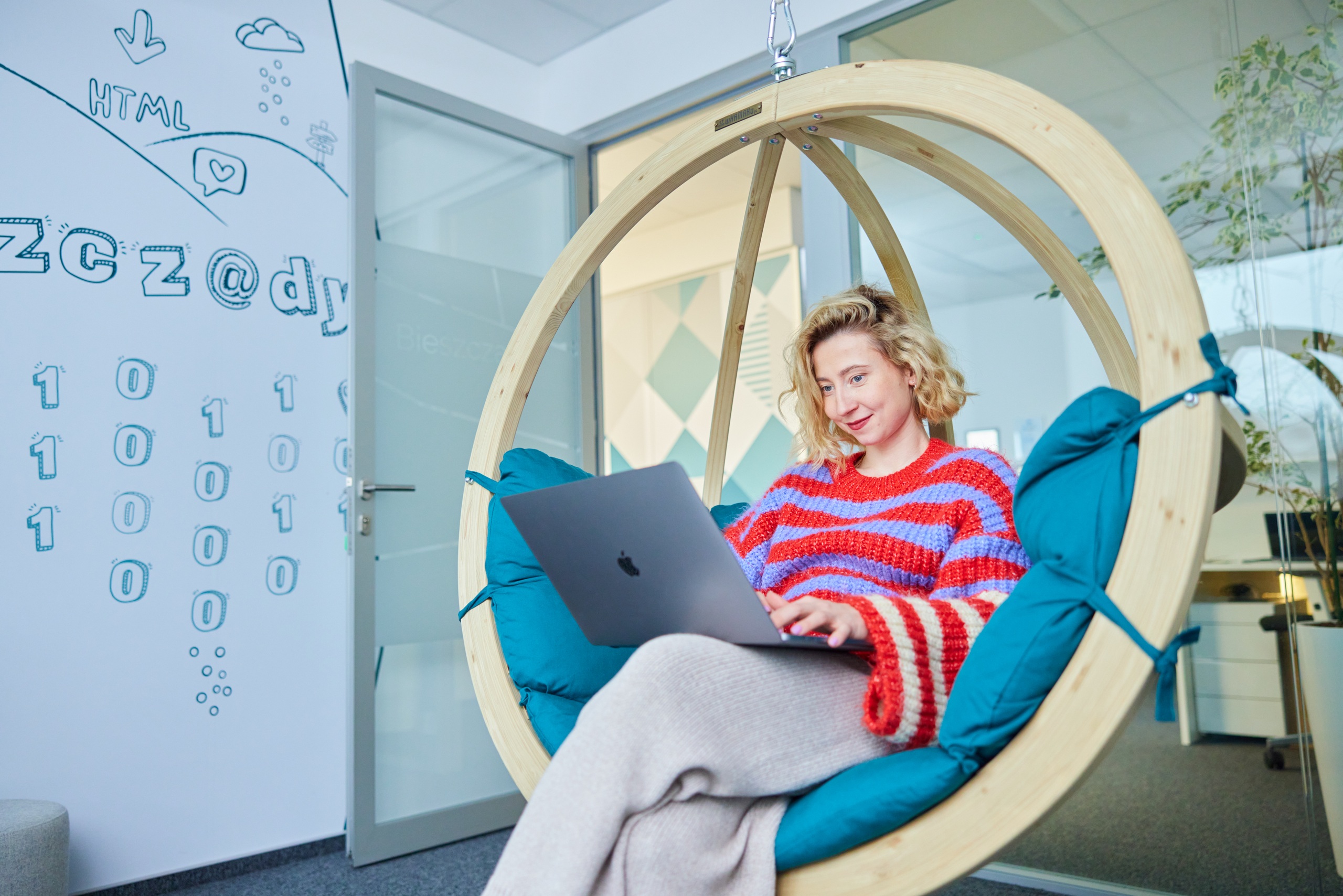
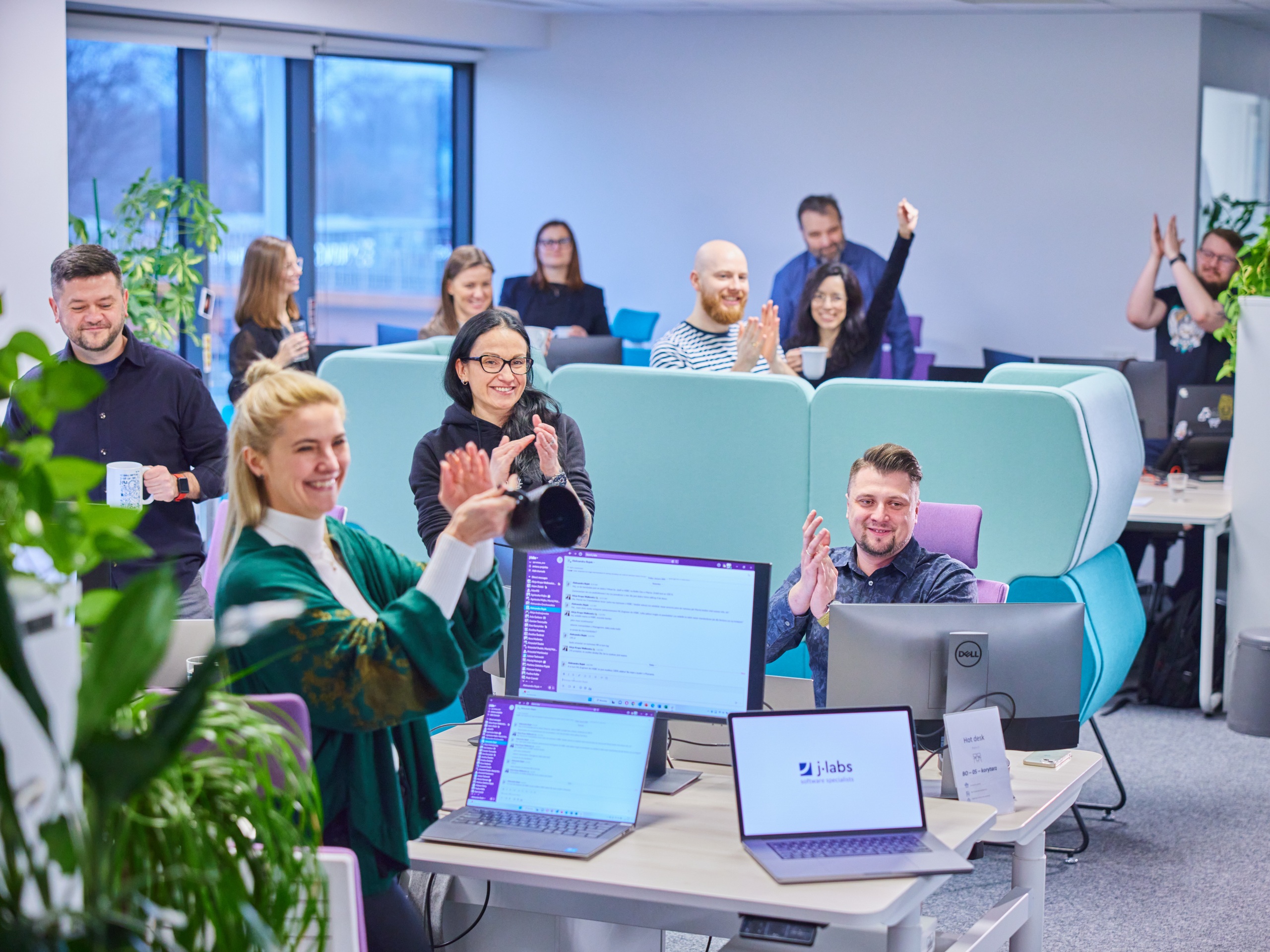
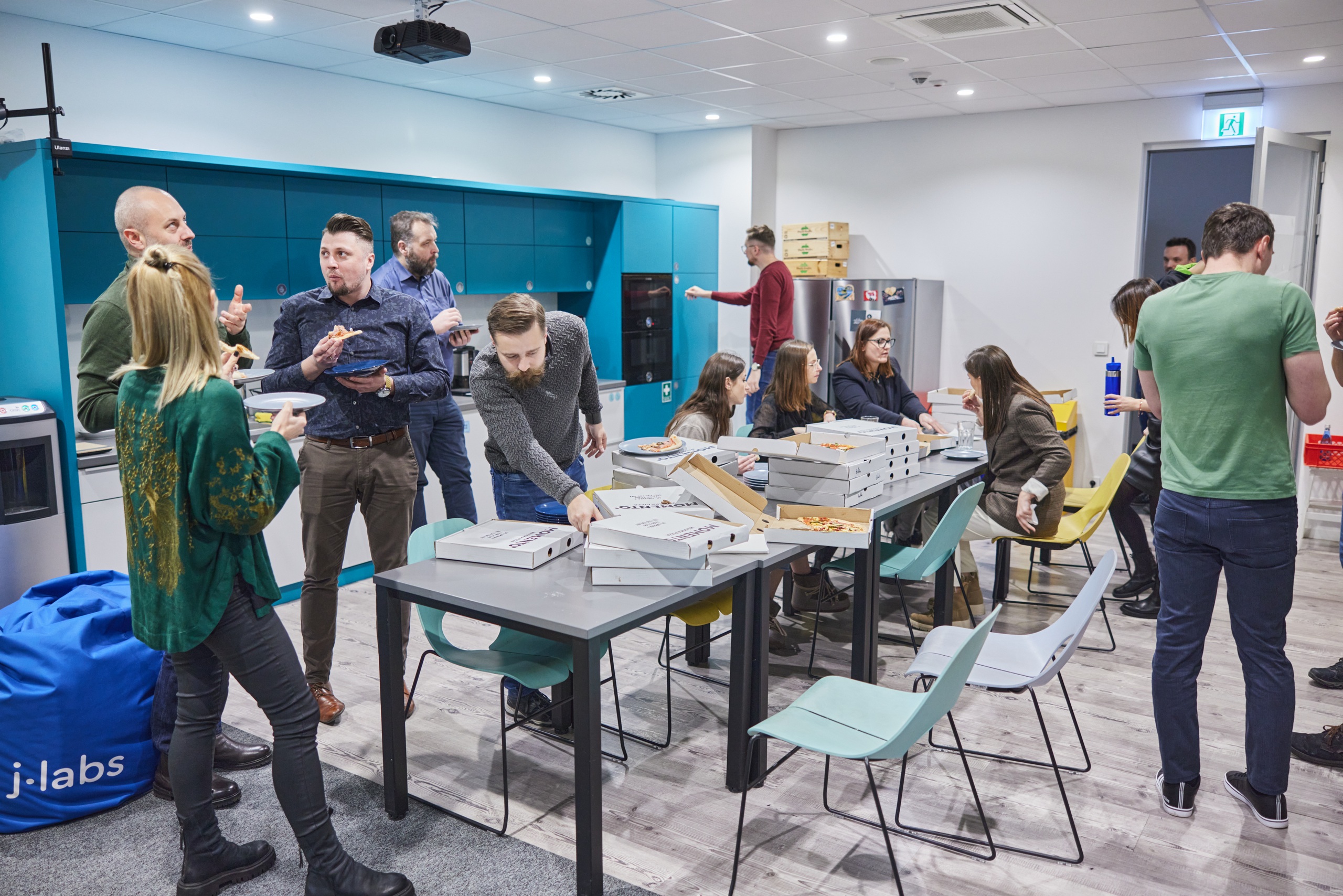
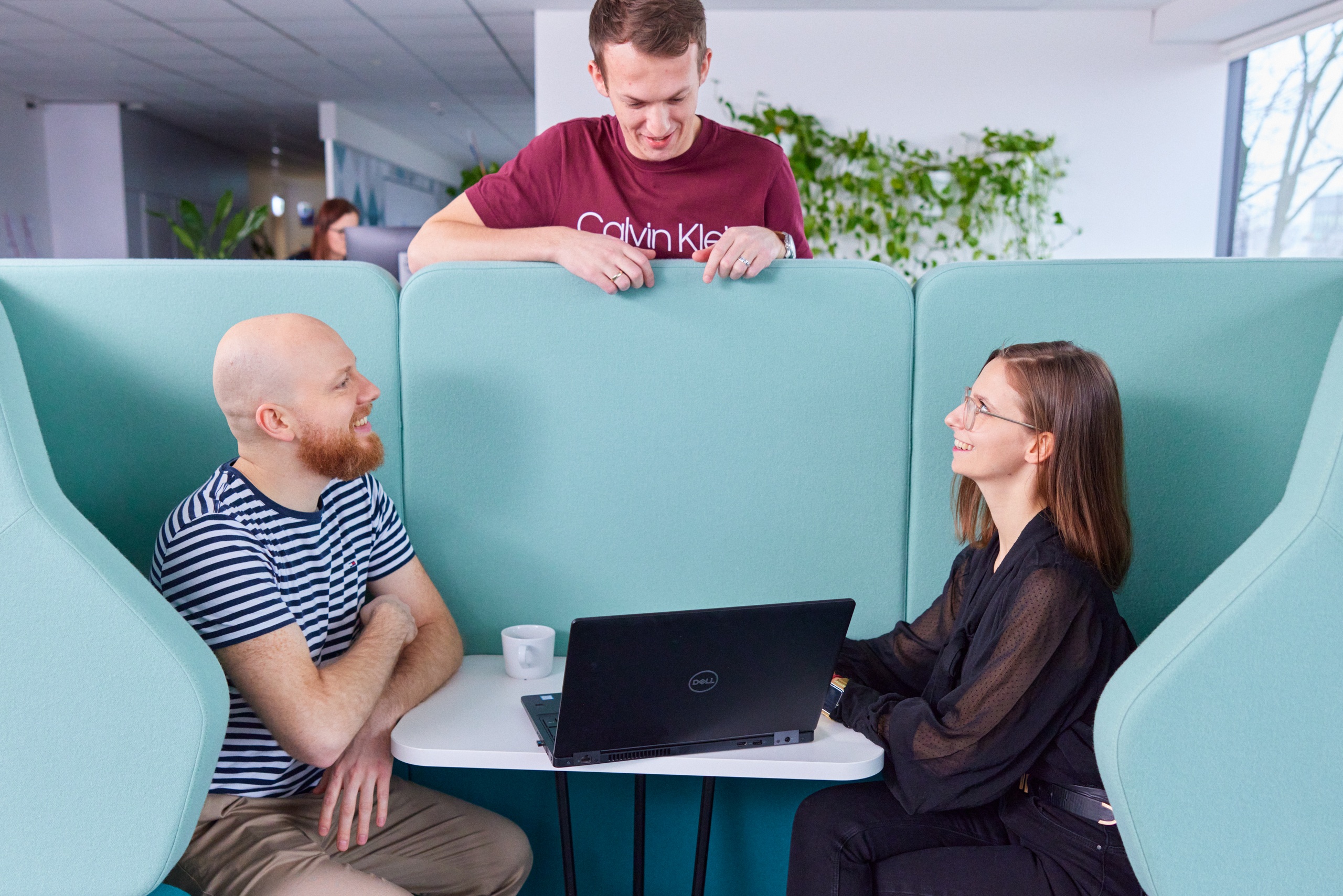